颜色、形状和纹理:使用 OpenCV 进行特征提取
点击上方“小白学视觉”,选择加"星标"或“置顶” 重磅干货,第一时间送达 ![]()
图像处理所做的只是从图像中提取有用的信息,从而减少数据量,但保留描述图像特征的像素。
1. 颜色
-
色调:描述主波长,是指定颜色的通道 -
饱和度:描述色调/颜色的纯度/色调 -
值:描述颜色的强度
import cv2
from google.colab.patches import cv2_imshow
image = cv2.imread(image_file)
hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
cv2_imshow(hsv_image)
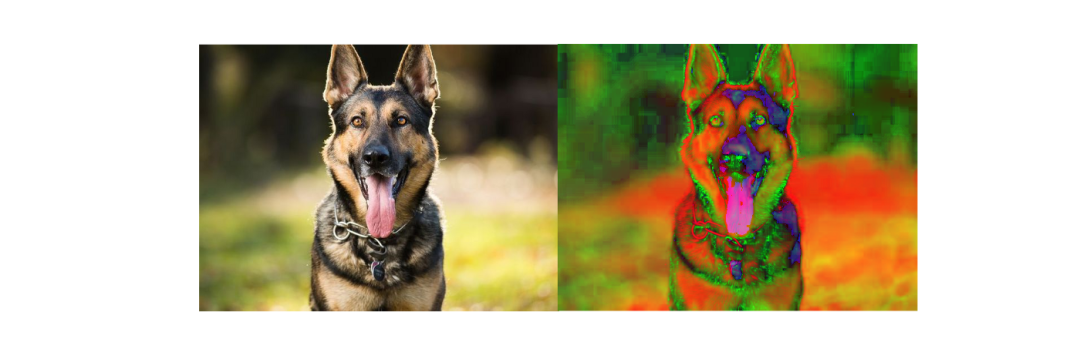
-
L:描述颜色的亮度,与强度互换使用 -
A : 颜色成分范围,从绿色到品红色 -
B:从蓝色到黄色的颜色分量
import cv2
from google.colab.patches import cv2_imshow
image = cv2.imread(image_file)
lab_image = cv2.cvtColor(image, cv2.COLOR_BGR2LAB)
cv2_imshow(lab_image)
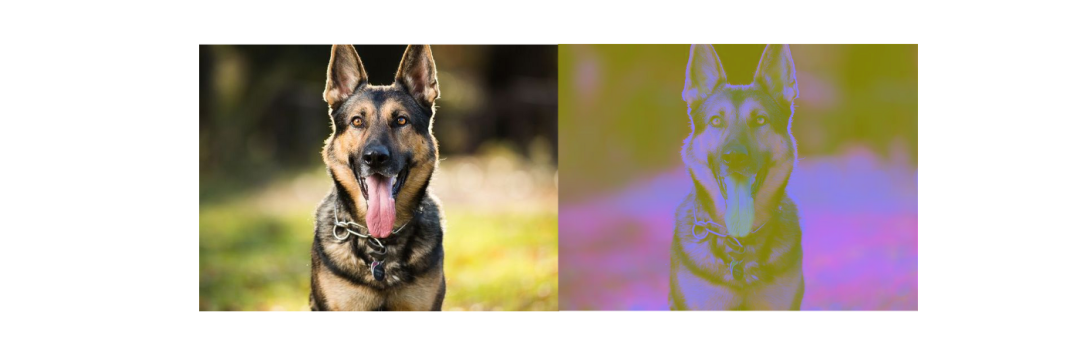
-
Y : 伽马校正后从 RGB 颜色空间获得的亮度 -
Cr:描述红色 (R) 分量与亮度的距离 -
Cb:描述蓝色 (B) 分量与亮度的距离
import cv2
from google.colab.patches import cv2_imshow
image = cv2.imread(image_file)
ycrcb_image = cv2.cvtColor(image, cv2.COLOR_BGR2YCrCb)
cv2_imshow(ycrcb_image)
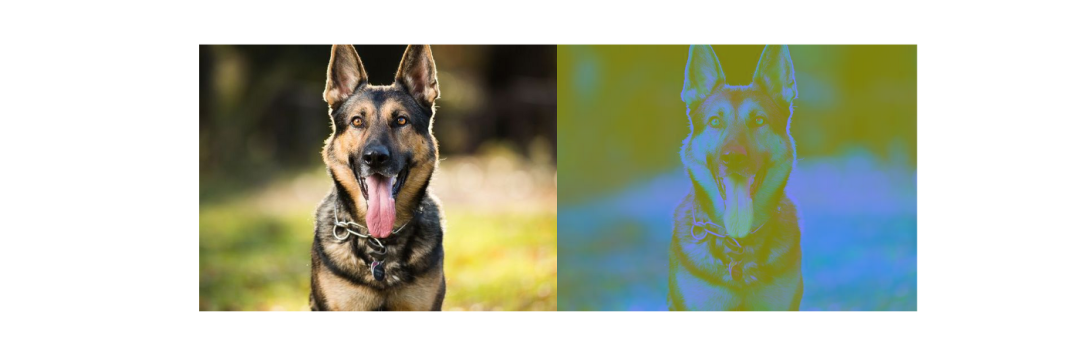
import cv2
from google.colab.patches import cv2_imshow
import numpy as np
import plotly.figure_factory as ff
# Check the distribution of red values
red_values = []
for i in range(len(images)):
red_value = np.mean(images[i][:, :, 0])
red_values.append(red_value)
# Check the distribution of green values
green_values = []
for i in range(len(images)):
green_value = np.mean(images[i][:, :, 1])
green_values.append(green_value)
# Check the distribution of blue values
blue_values = []
for i in range(len(images)):
blue_value = np.mean(images[i][:, :, 2])
blue_values.append(blue_value)
# Plotting the histogram
fig = ff.create_distplot([red_values, green_values, blue_values], group_labels=["R", "G", "B"], colors=['red', 'green', 'blue'])
fig.update_layout(showlegend=True, template="simple_white")
fig.update_layout(title_text='Distribution of channel values across images in RGB')
fig.data[0].marker.line.color = 'rgb(0, 0, 0)'
fig.data[0].marker.line.width = 0.5
fig.data[1].marker.line.color = 'rgb(0, 0, 0)'
fig.data[1].marker.line.width = 0.5
fig.data[2].marker.line.color = 'rgb(0, 0, 0)'
fig.data[2].marker.line.width = 0.5
fig
import cv2
from google.colab.patches import cv2_imshow
# Reading the original image
image_spot = cv2.imread(image_file)
cv2_imshow(image_spot)
# Converting it to HSV color space
hsv_image_spot = cv2.cvtColor(image_spot, cv2.COLOR_BGR2HSV)
cv2_imshow(hsv_image_spot)
# Setting the black pixel mask and perform bitwise_and to get only the black pixels
mask = cv2.inRange(hsv_image_spot, (0, 0, 0), (180, 255, 40))
masked = cv2.bitwise_and(hsv_image_spot, hsv_image_spot, mask=mask)
cv2_imshow(masked)
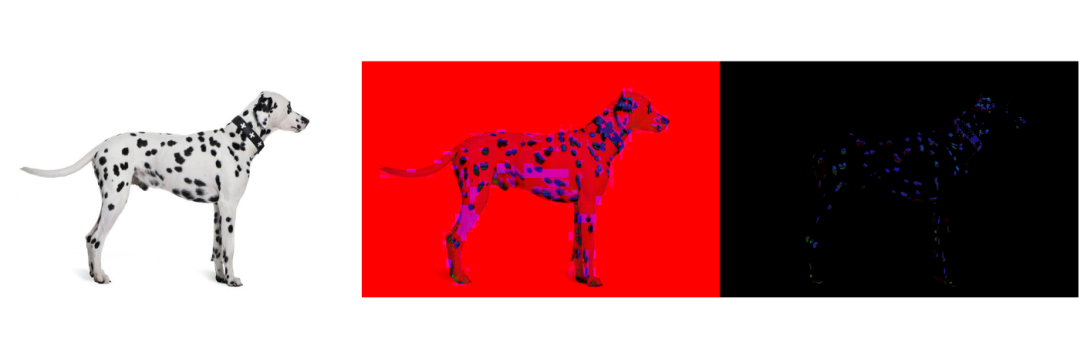
import cv2
from google.colab.patches import cv2_imshow
image_spot_reshaped = image_spot.reshape((image_spot.shape[0] * image_spot.shape[1], 3))
# convert to np.float32
Z = np.float32(image_spot_reshaped)
# define criteria, number of clusters(K) and apply kmeans()
criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 10, 1.0)
K = 2
ret, label, center = cv2.kmeans(Z, K, None, criteria, 10, cv2.KMEANS_RANDOM_CENTERS)
# Now convert back into uint8, and make original image
center = np.uint8(center)
res = center[label.flatten()]
res2 = res.reshape((image_spot.shape))
cv2_imshow(res2)
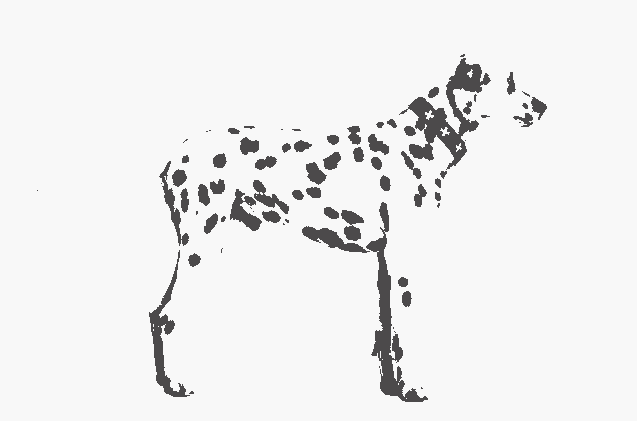
2. 形状
-
矩 -
轮廓面积 -
轮廓周长 -
轮廓近似 -
凸包 -
凸性检测 -
矩形边界 -
最小外接圆 -
拟合椭圆 -
拟合直线
3. 纹理
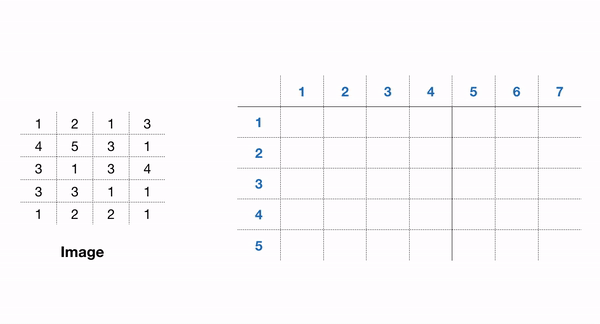
-
对比度:测量灰度共生矩阵的局部变化。 -
相关性:测量指定像素对的联合概率出现。 -
平方:提供 GLCM 中元素的平方和。也称为均匀性或角二阶矩。 -
同质性:测量 GLCM 中元素分布与 GLCM 对角线的接近程度。
import cv2
from google.colab.patches import cv2_imshow
image_spot = cv2.imread(image_file)
gray = cv2.cvtColor(image_spot, cv2.COLOR_BGR2GRAY)
# Find the GLCM
import skimage.feature as feature
# Param:
# source image
# List of pixel pair distance offsets - here 1 in each direction
# List of pixel pair angles in radians
graycom = feature.greycomatrix(gray, [1], [0, np.pi/4, np.pi/2, 3*np.pi/4], levels=256)
# Find the GLCM properties
contrast = feature.greycoprops(graycom, 'contrast')
dissimilarity = feature.greycoprops(graycom, 'dissimilarity')
homogeneity = feature.greycoprops(graycom, 'homogeneity')
energy = feature.greycoprops(graycom, 'energy')
correlation = feature.greycoprops(graycom, 'correlation')
ASM = feature.greycoprops(graycom, 'ASM')
print("Contrast: {}".format(contrast))
print("Dissimilarity: {}".format(dissimilarity))
print("Homogeneity: {}".format(homogeneity))
print("Energy: {}".format(energy))
print("Correlation: {}".format(correlation))
print("ASM: {}".format(ASM))
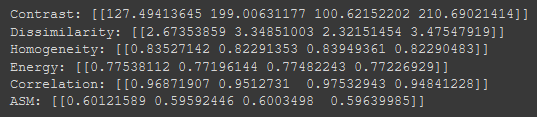
-
https://www.pyimagesearch.com/2015/12/07/local-binary-patterns-with-python-opencv/ -
https://towardsdatascience.com/face-recognition-how-lbph-works-90ec258c3d6b
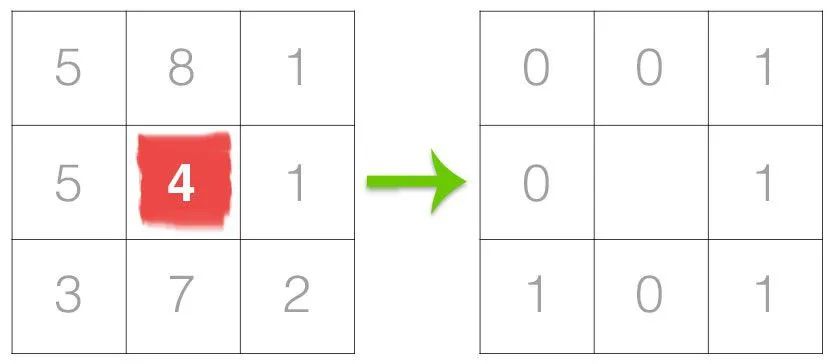
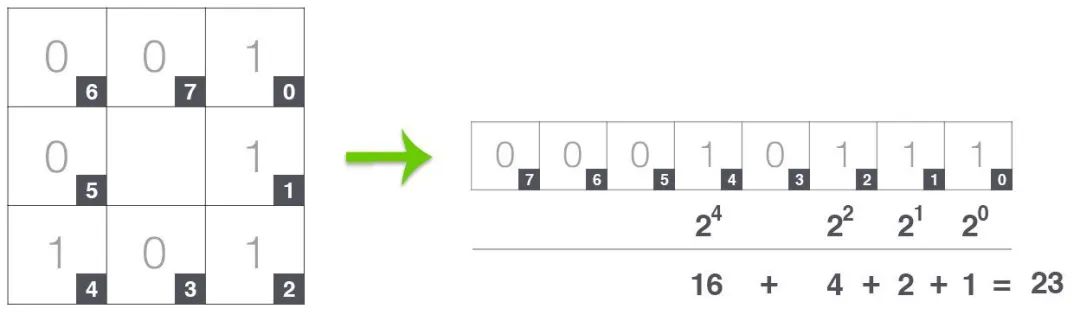
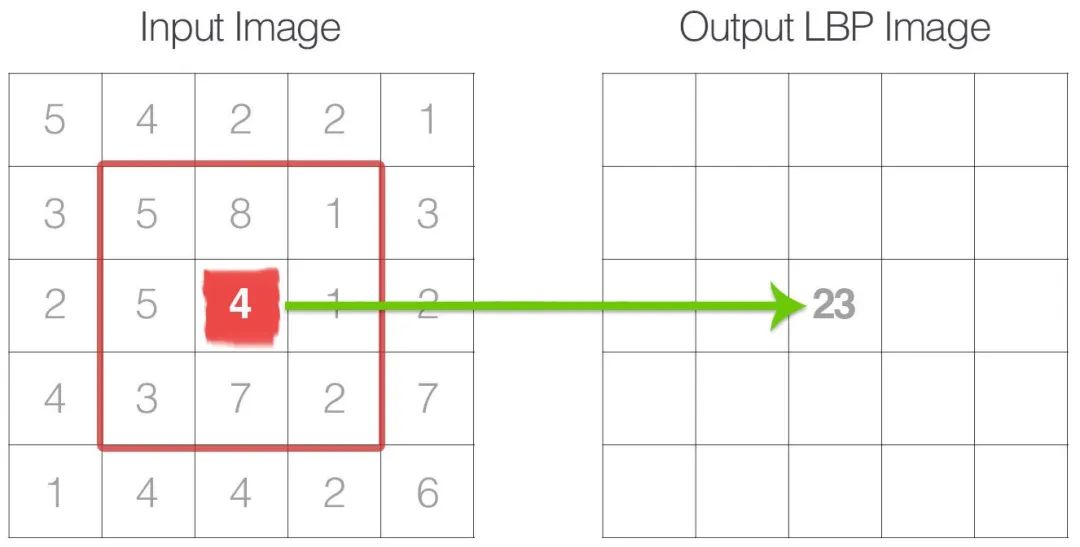
import cv2
from google.colab.patches import cv2_imshow
class LocalBinaryPatterns:
def __init__(self, numPoints, radius):
self.numPoints = numPoints
self.radius = radius
def describe(self, image, eps = 1e-7):
lbp = feature.local_binary_pattern(image, self.numPoints, self.radius, method="uniform")
(hist, _) = np.histogram(lbp.ravel(), bins=np.arange(0, self.numPoints+3), range=(0, self.numPoints + 2))
# Normalize the histogram
hist = hist.astype('float')
hist /= (hist.sum() + eps)
return hist, lbp
image = cv2.imread(image_file)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
desc = LocalBinaryPatterns(24, 8)
hist, lbp = desc.describe(gray)
print("Histogram of Local Binary Pattern value: {}".format(hist))
contrast = contrast.flatten()
dissimilarity = dissimilarity.flatten()
homogeneity = homogeneity.flatten()
energy = energy.flatten()
correlation = correlation.flatten()
ASM = ASM.flatten()
hist = hist.flatten()
features = np.concatenate((contrast, dissimilarity, homogeneity, energy, correlation, ASM, hist), axis=0)
cv2_imshow(gray)
cv2_imshow(lbp)
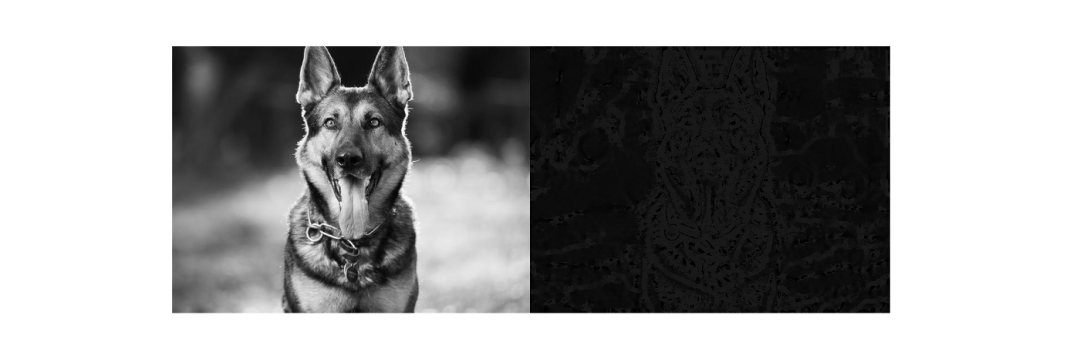
结论
参考
-
https://www.analyticsvidhya.com/blog/2019/08/3-techniques-extract-features-from-image-data-machine-learning-python/ -
https://www.mygreatlearning.com/blog/feature-extraction-in-image-processing/ -
https://thevatsalsaglani.medium.com/feature-extraction-from-medical-images-and-an-introduction-to-xtract-features-9a225243e94b -
http://www.scholarpedia.org/article/Local_Binary_Patterns -
https://medium.com/mlearning-ai/mlearning-ai-submission-suggestions-b51e2b130bfb
下载1:OpenCV-Contrib扩展模块中文版教程
在「小白学视觉」公众号后台回复:扩展模块中文教程,即可下载全网第一份OpenCV扩展模块教程中文版,涵盖扩展模块安装、SFM算法、立体视觉、目标跟踪、生物视觉、超分辨率处理等二十多章内容。
下载2:Python视觉实战项目52讲 在「小白学视觉」公众号后台回复:Python视觉实战项目,即可下载包括图像分割、口罩检测、车道线检测、车辆计数、添加眼线、车牌识别、字符识别、情绪检测、文本内容提取、面部识别等31个视觉实战项目,助力快速学校计算机视觉。
下载3:OpenCV实战项目20讲 在「小白学视觉」公众号后台回复:OpenCV实战项目20讲,即可下载含有20个基于OpenCV实现20个实战项目,实现OpenCV学习进阶。
交流群
欢迎加入公众号读者群一起和同行交流,目前有SLAM、三维视觉、传感器、自动驾驶、计算摄影、检测、分割、识别、医学影像、GAN、算法竞赛等微信群(以后会逐渐细分),请扫描下面微信号加群,备注:”昵称+学校/公司+研究方向“,例如:”张三 + 上海交大 + 视觉SLAM“。请按照格式备注,否则不予通过。添加成功后会根据研究方向邀请进入相关微信群。请勿在群内发送广告,否则会请出群,谢谢理解~
评论