一些你可能不知道的 Python 小技巧!
Python网络爬虫与数据挖掘
共 8158字,需浏览 17分钟
·
2021-03-04 17:46
文章转自:CSDN 参考链接:
https://levelup.gitconnected.com/python-tricks-i-can-not-live-without-87ae6aff3af8
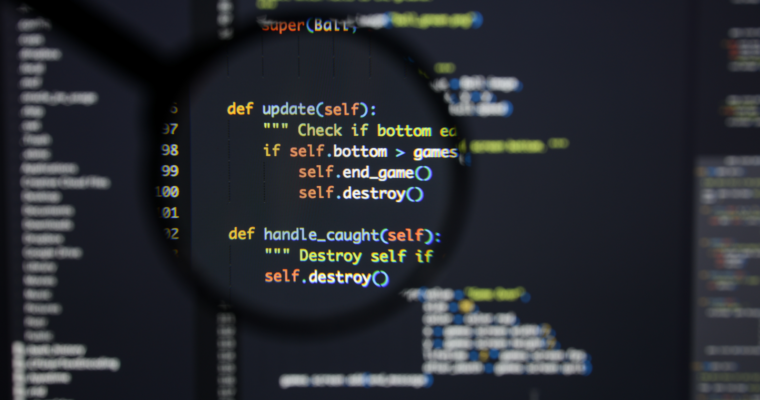
集合
myword = "NanananaBatman"
set(myword)
{'N', 'm', 'n', 'B', 'a', 't'}
# first you can easily change set to list and other way around
mylist = ["a", "b", "c","c"]
# let's make a set out of it
myset = set(mylist)
# myset will be:
{'a', 'b', 'c'}
# and, it's already iterable so you can do:
for element in myset:
print(element)
# but you can also convert it to list again:
mynewlist = list(myset)
# and mynewlist will be:
['a', 'b', 'c']
mylist = ["c", "c", "a","b"]
mynewlist = list(set(mylist))
# mynewlist is:
['a', 'b', 'c']
# this is the set of permissions before change;
original_permission_set = {"is_admin","can_post_entry", "can_edit_entry", "can_view_settings"}
# this is new set of permissions;
new_permission_set = {"can_edit_settings","is_member", "can_view_entry", "can_edit_entry"}
# now permissions to add will be:
new_permission_set.difference(original_permission_set)
# which will result:
{'can_edit_settings', 'can_view_entry', 'is_member'}
# As you can see can_edit_entry is in both sets; so we do notneed
# to worry about handling it
# now permissions to remove will be:
original_permission_set.difference(new_permission_set)
# which will result:
{'is_admin', 'can_view_settings', 'can_post_entry'}
# and basically it's also true; we switched admin to member, andadd
# more permission on settings; and removed the post_entrypermission
日历
import calendar
calendar.monthrange(2020, 12)
# will result:
(1, 31)
# BUT! you need to be careful here, why? Let's read thedocumentation:
help(calendar.monthrange)
# Help on function monthrange in module calendar:
# monthrange(year, month)
# Return weekday (0-6~ Mon-Sun) and number of days (28-31) for
# year, month.
# As you can see the first value returned in tuple is a weekday,
# not the number of the first day for a given month; let's try
# to get the same for 2021
calendar.monthrange(2021, 12)
(2, 31)
# So this basically means that the first day of December 2021 isWed
# and the last day of December 2021 is 31 (which is obvious,cause
# December always has 31 days)
# let's play with February
calendar.monthrange(2021, 2)
(0, 28)
calendar.monthrange(2022, 2)
(1, 28)
calendar.monthrange(2023, 2)
(2, 28)
calendar.monthrange(2024, 2)
(3, 29)
calendar.monthrange(2025, 2)
(5, 28)
# as you can see it handled nicely the leap year;
2)
29)
# means that February 2024 starts on Thursday
# let's define simple helper:
weekdays = ["Monday", "Tuesday","Wednesday", "Thursday", "Friday","Saturday", "Sunday"]
# now we can do something like:
weekdays[3]
# will result in:
'Thursday'
# now simple math to tell what day is 15th of February 2020:
offset = 3 # it's thefirst value from monthrange
for day in range(1, 29):
+ offset - 1) % 7])
1 Thursday
2 Friday
3 Saturday
4 Sunday
...
18 Sunday
19 Monday
20 Tuesday
21 Wednesday
22 Thursday
23 Friday
24 Saturday
...
28 Wednesday
29 Thursday
# which basically makes sense;
from datetime import datetime
mydate = datetime(2024, 2, 15)
datetime.weekday(mydate)
# will result:
3
# or:
datetime.strftime(mydate, "%A")
'Thursday'
# checking if year is leap:
calendar.isleap(2021) #False
calendar.isleap(2024) #True
# or checking how many days will be leap days for given yearspan:
calendar.leapdays(2021, 2026) # 1
calendar.leapdays(2020, 2026) # 2
# read the help here, as range is: [y1, y2), meaning that second
# year is not included;
calendar.leapdays(2020, 2024) # 1
枚举有第二个参数
mylist = ['a', 'b', 'd', 'c', 'g', 'e']
for i, item in enumerate(mylist):
item)
# Will give:
0 a
1 b
2 d
3 c
4 g
5 e
# but, you can add a start for enumeration:
for i, item in enumerate(mylist, 16):
item)
# and now you will get:
16 a
17 b
18 d
19 c
20 g
21 e
if-else 逻辑
OPEN = 1
IN_PROGRESS = 2
CLOSED = 3
def handle_open_status():
print('Handling openstatus')
def handle_in_progress_status():
print('Handling inprogress status')
def handle_closed_status():
print('Handling closedstatus')
def handle_status_change(status):
if status == OPEN:
handle_open_status()
elif status ==IN_PROGRESS:
handle_in_progress_status()
elif status == CLOSED:
handle_closed_status()
handle_status_change(1) #Handling open status
handle_status_change(2) #Handling in progress status
handle_status_change(3) #Handling closed status
from enum import IntEnum
class StatusE(IntEnum):
OPEN = 1
IN_PROGRESS = 2
CLOSED = 3
def handle_open_status():
print('Handling openstatus')
def handle_in_progress_status():
print('Handling inprogress status')
def handle_closed_status():
print('Handling closedstatus')
handlers = {
StatusE.OPEN.value:handle_open_status,
StatusE.IN_PROGRESS.value: handle_in_progress_status,
StatusE.CLOSED.value:handle_closed_status
}
def handle_status_change(status):
if status not inhandlers:
raiseException(f'No handler found for status: {status}')
handler =handlers[status]
handler()
handle_status_change(StatusE.OPEN.value) # Handling open status
handle_status_change(StatusE.IN_PROGRESS.value) # Handling in progress status
handle_status_change(StatusE.CLOSED.value) # Handling closed status
handle_status_change(4) #Will raise the exception
enum 模块
from enum import Enum, IntEnum, Flag, IntFlag
class MyEnum(Enum):
FIRST ="first"
SECOND ="second"
THIRD ="third"
class MyIntEnum(IntEnum):
ONE = 1
TWO = 2
THREE = 3
# Now we can do things like:
MyEnum.FIRST #<MyEnum.FIRST: 'first'>
# it has value and name attributes, which are handy:
MyEnum.FIRST.value #'first'
MyEnum.FIRST.name #'FIRST'
# additionally we can do things like:
MyEnum('first') #<MyEnum.FIRST: 'first'>, get enum by value
MyEnum['FIRST'] #<MyEnum.FIRST: 'first'>, get enum by name
MyEnum.FIRST == "first" # False
# but
MyIntEnum.ONE == 1 # True
# to make first example to work:
MyEnum.FIRST.value == "first" # True
from enum import Enum
from django.utils.translation import gettext_lazy as _
class MyEnum(Enum):
FIRST ="first"
SECOND ="second"
THIRD ="third"
def choices(cls):
return [
(cls.FIRST.value, _('first')),
(cls.SECOND.value, _('second')),
(cls.THIRD.value, _('third'))
]
# And later in eg. model definiton:
some_field = models.CharField(max_length=10,choices=MyEnum.choices())
iPython
pip install ipython
# you should see something like this after you start:
Python 3.8.5 (default, Jul 28 2020, 12:59:40)
Type 'copyright', 'credits' or 'license' for more information
IPython 7.18.1 -- An enhanced Interactive Python. Type '?' forhelp.
In [1]:
(完)
回复关键字“简明python ”,立即获取入门必备书籍《简明python教程》电子版
回复关键字“爬虫”,立即获取爬虫学习资料
python入门与进阶 每天与你一起成长 推荐阅读
评论