python“不为人知的”特性
Python日志
共 8250字,需浏览 17分钟
·
2021-06-01 22:52

>>> x = 5
>>> 1 < x < 10
True
>>> 10 < x < 20
False
>>> x < 10 < x*10 < 100
True
>>> 10 > x <= 9
True
>>> 5 == x > 4
True
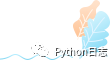
>>> a = ['a', 'b', 'c', 'd', 'e']
>>> for index, item in enumerate(a): print index, item
...
0 a
1 b
2 c
3 d
4 e
>>>
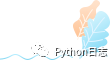
for i in range(len(a)):
print i, a[i]
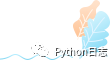
x=(n for n in foo if bar(n)) #foo是可迭代对象
>>> type(x)
<type 'generator'>
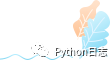
for n in x:
pass
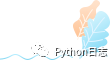
x = [n for n in foo if bar(n)]
>>> type(x)
<type 'list'>
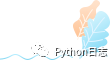
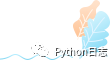
iter(collection)---> iterator
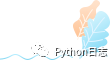
iter(callable, sentinel) --> iterator
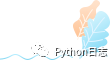
def seek_next_line(f):
#每次读一个字符,直到出现换行符就返回
for c in iter(lambda: f.read(1),'\n'):
pass
>>> def foo(x=[]):
... x.append(1)
... print x
...
>>> foo()
[1]
>>> foo()
[1, 1]
>>> foo()
[1, 1, 1]
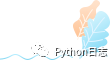
>>> def foo(x=None):
... if x is None:
... x = []
... x.append(1)
... print x
>>> foo()
[1]
>>> foo()
[1]
def mygen():
"""Yield 5 until something else is passed back via send()"""
a = 5
while True:
f = (yield a) #yield a and possibly get f in return
if f is not None:
a = f #store the new value
你可以:
>>> g = mygen()
>>> g.next()
5
>>> g.next()
5
>>> g.send(7) #we send this back to the generator
7
>>> g.next() #now it will yield 7 until we send something else
7
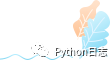
>>> from __future__ import braces #这里的braces 指的是:curly braces(花括号)
File "<stdin>", line 1
SyntaxError: not a chance
当然这仅仅是一个玩笑,想用花括号定义函数?没门。感兴趣的还可以了解下:
from __future__ import barry_as_FLUFL
不过这是python3里面的特性
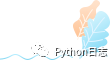
a = [1,2,3,4,5]
>>> a[::2] # iterate over the whole list in 2-increments
[1,3,5]
还有一个特例: x[::-1]
,反转列表:
>>> a[::-1]
[5,4,3,2,1]
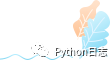
>>> l = range(5)
>>> l
[0, 1, 2, 3, 4]
>>> l.reverse()
>>> l
[4, 3, 2, 1, 0]
>>> l2 = reversed(l)
>>> l2
<listreverseiterator object at 0x99faeec>
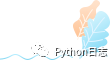
>>> def print_args(function):
>>> def wrapper(*args, **kwargs):
>>> print 'Arguments:', args, kwargs
>>> return function(*args, **kwargs)
>>> return wrapper
>>> @print_args
>>> def write(text):
>>> print text
>>> write('foo')
Arguments: ('foo',) {}
foo
@是语法糖,它等价于:
>>> write = print_args(write)
>>> write('foo')
arguments: ('foo',) {}
foo
for i in foo:
if i == 0:
break
else:
print("i was never 0")
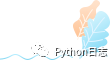
found = False
for i in foo:
if i == 0:
found = True
break
if not found:
print("i was never 0")
python2.5有个 __missing__ 方法
dict的子类如果定义了方法 __missing__(self, key) ,如果key不再dict中,那么d[key]就会调用 __missing__ 方法,而且d[key]的返回值就是 __missing__ 的返回值。
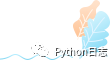
>>> class MyDict(dict):
... def __missing__(self, key):
... self[key] = rv = []
... return rv
...
>>> m = MyDict()
>>> m["foo"].append(1)
>>> m["foo"].append(2)
>>> dict(m)
{'foo': [1, 2]}
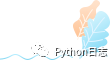
>>> from collections import defaultdict
>>> m = defaultdict(list)
>>> m["foo"].append(1)
>>> m["foo"].append(2)
>>> dict(m)
{'foo': [1, 2]}
>>> a = 10
>>> b = 5
>>> a, b
(10, 5)
>>> a, b = b, a
>>> a, b
(5, 10)
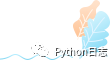
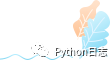
>>> pattern = """
... ^ # beginning of string
... M{0,4} # thousands - 0 to 4 M's
... (CM|CD|D?C{0,3}) # hundreds - 900 (CM), 400 (CD), 0-300 (0 to 3 C's),
... # or 500-800 (D, followed by 0 to 3 C's)
... (XC|XL|L?X{0,3}) # tens - 90 (XC), 40 (XL), 0-30 (0 to 3 X's),
... # or 50-80 (L, followed by 0 to 3 X's)
... (IX|IV|V?I{0,3}) # ones - 9 (IX), 4 (IV), 0-3 (0 to 3 I's),
... # or 5-8 (V, followed by 0 to 3 I's)
... $ # end of string
... """
>>> re.search(pattern, 'M', re.VERBOSE)
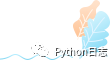
def draw_point(x, y):
# do some magic
point_foo = (3, 4)
point_bar = {'y': 3, 'x': 2}
draw_point(*point_foo)
draw_point(**point_bar)
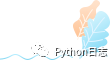
>>> NewType = type("NewType", (object,), {"x": "hello"})
>>> n = NewType()
>>> n.x
"hello"
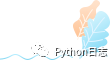
>>> class NewType(object):
>>> x = "hello"
>>> n = NewType()
>>> n.x
"hello"
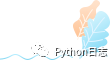
with open('foo.txt', 'w') as f:
f.write('hello!')
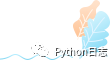
sum[value] = sum.get(value, 0) + 1
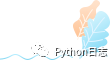
>>> d = {'key':123}
>>> d.setdefault('key',456)
123
>>> d['key']
123
>>> d.setdefault('key2',456)
456
>>> d['key2']
456
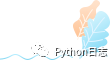
>>> cnt = Counter('helloworld')
>>> cnt
Counter({'l': 3, 'o': 2, 'e': 1, 'd': 1, 'h': 1, 'r': 1, 'w': 1})
>>> cnt['l']
3
>>> cnt['x'] = 10
>>> cnt.get('y')
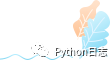
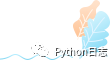
x = 3 if (y == 1) else 2
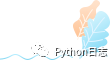
(func1 if y == 1 else func2)(arg1, arg2)
如果y等于1,那么调用func1(arg1,arg2)否则调用func2(arg1,arg2) x = (class1 if y == 1 else class2)(arg1, arg2)
try:
try_this(whatever)
except SomeException, exception:
#Handle exception
else:
# do something
finally:
#do something
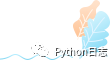
评论