HTTP客户端连接,选择HttpClient还是OkHttp?
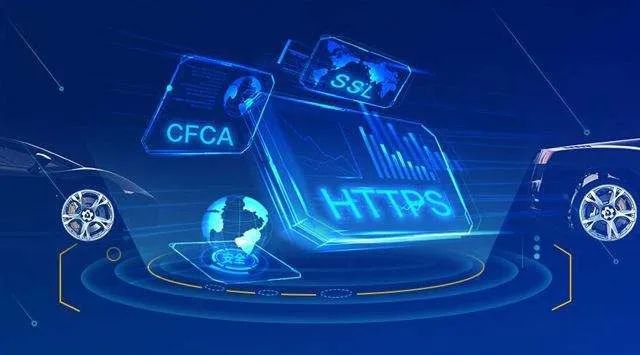
写在前面
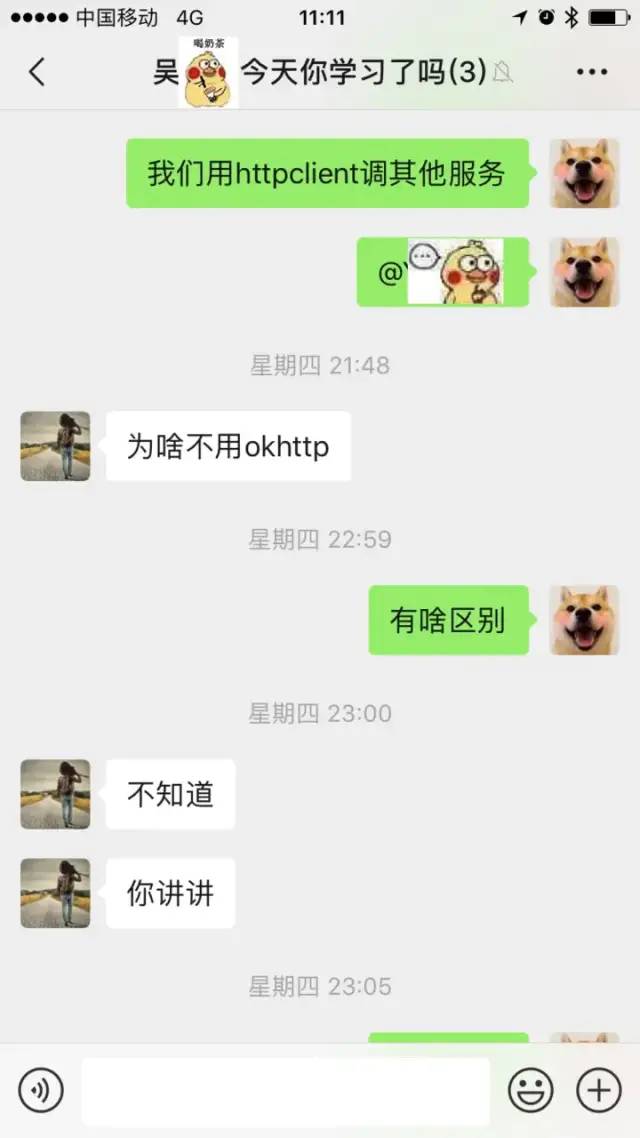
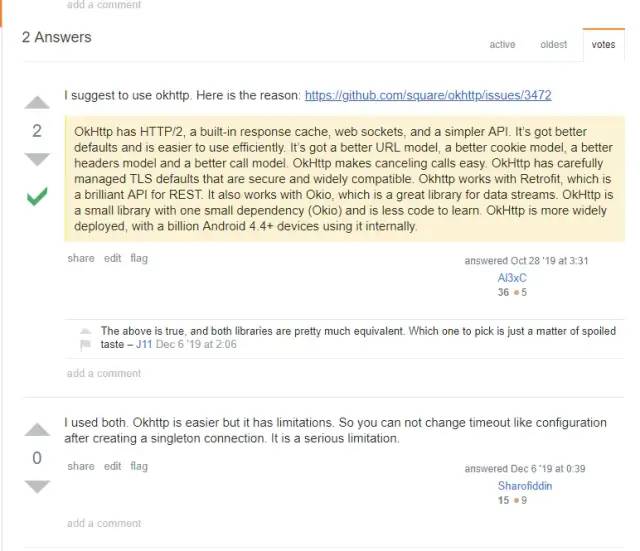
使用
HttpClient 使用介绍
创建 CloseableHttpClient 对象或 CloseableHttpAsyncClient 对象,前者同步,后者为异步 创建 Http 请求对象 调用 execute 方法执行请求,如果是异步请求在执行之前需调用 start 方法
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
@Test
public void testGet() throws IOException {
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
HttpGet httpGet = new HttpGet(url);
CloseableHttpResponse response = httpClient.execute(httpGet);
System.out.println(EntityUtils.toString(response.getEntity()));
}
@Test
public void testPut() throws IOException {
String api = "/api/user";
String url = String.format("%s%s", BASE\_URL, api);
HttpPut httpPut = new HttpPut(url);
UserVO userVO = UserVO.builder().name("h2t").id(16L).build();
httpPut.setHeader("Content-Type", "application/json;charset=utf8");
httpPut.setEntity(new StringEntity(JSONObject.toJSONString(userVO), "UTF-8"));
CloseableHttpResponse response = httpClient.execute(httpPut);
System.out.println(EntityUtils.toString(response.getEntity()));
}
添加对象
@Test
public void testPost() throws IOException {
String api = "/api/user";
String url = String.format("%s%s", BASE\_URL, api);
HttpPost httpPost = new HttpPost(url);
UserVO userVO = UserVO.builder().name("h2t2").build();
httpPost.setHeader("Content-Type", "application/json;charset=utf8");
httpPost.setEntity(new StringEntity(JSONObject.toJSONString(userVO), "UTF-8"));
CloseableHttpResponse response = httpClient.execute(httpPost);
System.out.println(EntityUtils.toString(response.getEntity()));
}
上传文件
@Test
public void testUpload1() throws IOException {
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
HttpPost httpPost = new HttpPost(url);
File file = new File("C:/Users/hetiantian/Desktop/学习/docker\_practice.pdf");
FileBody fileBody = new FileBody(file);
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.setMode(HttpMultipartMode.BROWSER\_COMPATIBLE);
builder.addPart("file", fileBody); //addPart上传文件
HttpEntity entity = builder.build();
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
System.out.println(EntityUtils.toString(response.getEntity()));
}
@Test
public void testDelete() throws IOException {
String api = "/api/user/12";
String url = String.format("%s%s", BASE\_URL, api);
HttpDelete httpDelete = new HttpDelete(url);
CloseableHttpResponse response = httpClient.execute(httpDelete);
System.out.println(EntityUtils.toString(response.getEntity()));
}
@Test
public void testCancel() throws IOException {
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
HttpGet httpGet = new HttpGet(url);
httpGet.setConfig(requestConfig); //设置超时时间
//测试连接的取消
long begin = System.currentTimeMillis();
CloseableHttpResponse response = httpClient.execute(httpGet);
while (true) {
if (System.currentTimeMillis() - begin > 1000) {
httpGet.abort();
System.out.println("task canceled");
break;
}
}
System.out.println(EntityUtils.toString(response.getEntity()));
}
task canceled
cost 8098 msc
Disconnected from the target VM, address: '127.0.0.1:60549', transport: 'socket'
java.net.SocketException: socket closed...【省略】
OkHttp 使用
创建 OkHttpClient 对象 创建 Request 对象 将 Request 对象封装为 Call 通过 Call 来执行同步或异步请求,调用 execute 方法同步执行,调用 enqueue 方法异步执行
private OkHttpClient client = new OkHttpClient();
@Test
public void testGet() throws IOException {
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
Request request = new Request.Builder()
.url(url)
.get()
.build();
final Call call = client.newCall(request);
Response response = call.execute();
System.out.println(response.body().string());
}
@Test
public void testPut() throws IOException {
String api = "/api/user";
String url = String.format("%s%s", BASE\_URL, api);
//请求参数
UserVO userVO = UserVO.builder().name("h2t").id(11L).build();
RequestBody requestBody = RequestBody.create(MediaType.parse("application/json; charset=utf-8"),
JSONObject.toJSONString(userVO));
Request request = new Request.Builder()
.url(url)
.put(requestBody)
.build();
final Call call = client.newCall(request);
Response response = call.execute();
System.out.println(response.body().string());
}
添加对象
@Test
public void testPost() throws IOException {
String api = "/api/user";
String url = String.format("%s%s", BASE\_URL, api);
//请求参数
JSONObject json = new JSONObject();
json.put("name", "hetiantian");
RequestBody requestBody = RequestBody.create(MediaType.parse("application/json; charset=utf-8"), String.valueOf(json));
Request request = new Request.Builder()
.url(url)
.post(requestBody) //post请求
.build();
final Call call = client.newCall(request);
Response response = call.execute();
System.out.println(response.body().string());
}
上传文件
@Test
public void testUpload() throws IOException {
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
RequestBody requestBody = new MultipartBody.Builder()
.setType(MultipartBody.FORM)
.addFormDataPart("file", "docker\_practice.pdf",
RequestBody.create(MediaType.parse("multipart/form-data"),
new File("C:/Users/hetiantian/Desktop/学习/docker\_practice.pdf")))
.build();
Request request = new Request.Builder()
.url(url)
.post(requestBody) //默认为GET请求,可以不写
.build();
final Call call = client.newCall(request);
Response response = call.execute();
System.out.println(response.body().string());
}
@Test
public void testDelete() throws IOException {
String url = String.format("%s%s", BASE\_URL, api);
//请求参数
Request request = new Request.Builder()
.url(url)
.delete()
.build();
final Call call = client.newCall(request);
Response response = call.execute();
System.out.println(response.body().string());
}
@Test
public void testCancelSysnc() throws IOException {
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
Request request = new Request.Builder()
.url(url)
.get()
.build();
final Call call = client.newCall(request);
Response response = call.execute();
long start = System.currentTimeMillis();
//测试连接的取消
while (true) {
//1分钟获取不到结果就取消请求
if (System.currentTimeMillis() - start > 1000) {
call.cancel();
System.out.println("task canceled");
break;
}
}
System.out.println(response.body().string());
}
task canceled
cost 9110 msc
java.net.SocketException: socket closed...【省略】
小结
OkHttp 使用 build 模式创建对象来的更简洁一些,并且使用. post/.delete/.put/.get 方法表示请求类型,不需要像 HttpClient 创建 HttpGet、HttpPost 等这些方法来创建请求类型 依赖包上,如果 HttpClient 需要发送异步请求、实现文件上传,需要额外的引入异步请求依赖
<dependency>
<groupId>org.apache.httpcomponentsgroupId>
<artifactId>httpmimeartifactId>
<version>4.5.3version>
dependency>
<dependency>
<groupId>org.apache.httpcomponentsgroupId>
<artifactId>httpasyncclientartifactId>
<version>4.5.3version>
dependency>
请求的取消,HttpClient 使用 abort 方法,OkHttp 使用 cancel 方法,都挺简单的,如果使用的是异步 client,则在抛出异常时调用取消请求的方法即可
超时设置
private CloseableHttpClient httpClient = HttpClientBuilder.create().build();
private RequestConfig requestConfig = RequestConfig.custom()
.setSocketTimeout(60 \* 1000)
.setConnectTimeout(60 \* 1000).build();
String api = "/api/files/1";
String url = String.format("%s%s", BASE\_URL, api);
HttpGet httpGet = new HttpGet(url);
httpGet.setConfig(requestConfig); //设置超时时间
private OkHttpClient client = new OkHttpClient.Builder()
.connectTimeout(60, TimeUnit.SECONDS)//设置连接超时时间
.readTimeout(60, TimeUnit.SECONDS)//设置读取超时时间
.build();
HttpClient 和 OkHttp 性能比较
CPU 六核 内存 8G windows10
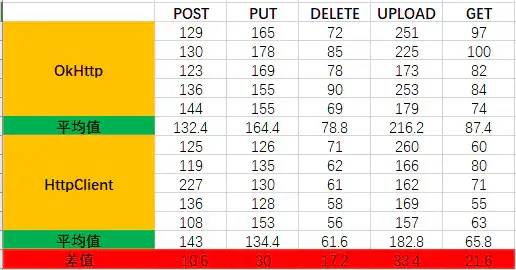
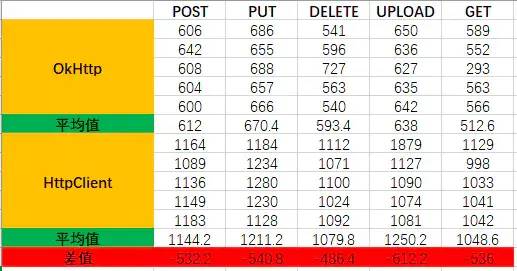
总结
最近热文
• 如果面试被问“红黑树”,可以这样回答 • 她是北大“一个人的毕业照”主人公,2010级古生物专业独苗,十年后搞起了AI • 刚刚用鸿蒙跑了个“hello world”!跑通后,我特么开始怀疑人生了.... • 今天终于搞懂了:为什么 Java 的 main 方法必须是 public static void? 最近整理了一份大厂算法刷题指南,包括一些刷题技巧,在知乎上已经有上万赞。同时还整理了一份6000页面试笔记。关注下面公众号,在公众号内回复「刷题」,即可免费获取!回复「加群」,可以邀请你加入读者群!
明天见(。・ω・。)ノ♡
评论