注意时间!用python制作倒计时提醒工具
Python客栈
共 4114字,需浏览 9分钟
·
2020-11-30 16:04
长时间的久坐容易疲劳,也容易腰背部肌肉容易受损,平时工作学习的时候我们应该时不时的起来活动一会,不如就用python制作个简单的倒计时提醒工具吧。
一、导入所需的模块
from tkinter import *
import time
from playsound import playsound
tkinter用来制作图形界面,time模块用来显示计算时间,playsound模块用来设置提示音。
二、利用tkinter制作窗口
root = Tk()
root.geometry('400x300')
root.resizable(0,0)
root.title('倒计时')
root.mainloop()
TK():初始化窗口
geometry():设置窗口的大小
resizable():锁定窗口大小
title():窗口标题
mainloop():始终显示窗口
三、创建当前时间
在窗口内创建一个标签 用来提示当前时间
Label(root, font ='arial 15 bold ', text = '当前时间:', bg = 'Pink').place(x = 40 ,y = 70)
font:字体参数
text:要显示的文本
bg:标签的背景颜色
place():标签的坐标位置
显示当前时间
def clock():
clock_time = time.strftime('%H:%M:%S %p')
curr_time.config(text = clock_time)
curr_time.after(1000,clock)
curr_time =Label(root, font ='arial 15 bold', text = '' , bg ='pink')
curr_time.place(x = 190 , y = 70)
clock()
strftime():用来格式化输出时间,%H为24小时制小时数,%M为分钟数,%s为秒数,%p用来提示a.m或p.m。
after():设置1秒的时间更新。
四、实现倒计时功能
创建倒计时的输入框
sec = StringVar()
Entry(root, textvariable = sec, width = 2, font = 'arial 12').place(x=250, y=155)
sec.set('00')
mins= StringVar()
Entry(root, textvariable = mins, width =2, font = 'arial 12').place(x=225, y=155)
mins.set('00')
hrs= StringVar()
Entry(root, textvariable = hrs, width =2, font = 'arial 12').place(x=200, y=155)
hrs.set('00')
sec,mins,hrs:存储秒,分,小时的字符串变量
Entry():创建文本输入框
textvariable:和一个特定变量绑定
倒计时功能
def countdown():
times = int(hrs.get())*3600+ int(mins.get())*60 + int(sec.get())
while times > -1:
minute,second = (times // 60 , times % 60)
hour = 0
if minute > 60:
hour , minute = (minute // 60 , minute % 60)
sec.set(second)
mins.set(minute)
hrs.set(hour)
root.update()
time.sleep(1)
if(times == 0):
playsound('Loud_Alarm_Clock_Buzzer.mp3')
sec.set('00')
mins.set('00')
hrs.set('00')
times -= 1
time变量获取总时间(小时*3600+分钟*60+秒数),time大于-1时,倒计时执行,当time=0时,调用提示音(同目录下的声音文件)。
补上倒计时前的标签
Label(root, font ='arial 15 bold', text = '倒计时:', bg ='pink').place(x = 40 ,y = 150)
五、创建一个按钮,启用倒计时
Button(root, text='START', bd ='10', command = countdown, bg = 'pink', font = 'arial 10 bold').place(x=150, y=210)
command:点击按钮时,调用上面编写好的倒计时函数
六、完整代码
讲解都在上面喽,就不写注释啦
from tkinter import *
import time
from playsound import playsound
root = Tk()
root.geometry('400x300')
root.resizable(0,0)
root.title('倒计时')
Label(root, font ='arial 15 bold ', text = '当前时间:', bg = 'Pink').place(x = 40 ,y = 70)
def clock():
clock_time = time.strftime('%H:%M:%S %p')
curr_time.config(text = clock_time)
curr_time.after(1000,clock)
curr_time =Label(root, font ='arial 15 bold', text = '' , bg ='pink')
curr_time.place(x = 190 , y = 70)
clock()
Label(root, font ='arial 15 bold', text = '倒计时', bg ='pink').place(x = 40 ,y = 150)
sec = StringVar()
Entry(root, textvariable = sec, width = 2, font = 'arial 12').place(x=250, y=155)
sec.set('00')
mins= StringVar()
Entry(root, textvariable = mins, width =2, font = 'arial 12').place(x=225, y=155)
mins.set('00')
hrs= StringVar()
Entry(root, textvariable = hrs, width =2, font = 'arial 12').place(x=200, y=155)
hrs.set('00')
def countdown():
times = int(hrs.get())*3600+ int(mins.get())*60 + int(sec.get())
while times > -1:
minute,second = (times // 60 , times % 60)
hour = 0
if minute > 60:
hour , minute = (minute // 60 , minute % 60)
sec.set(second)
mins.set(minute)
hrs.set(hour)
root.update()
time.sleep(1)
if(times == 0):
playsound('Loud_Alarm_Clock_Buzzer.mp3')
sec.set('00')
mins.set('00')
hrs.set('00')
times -= 1
Label(root, font ='arial 15 bold', text = '倒计时:', bg ='pink').place(x = 40 ,y = 150)
Button(root, text='START', bd ='10', command = countdown, bg = 'pink', font = 'arial 10 bold').place(x=150, y=210)
root.mainloop()

往期推荐
以上三位小伙伴,快来联系小编领取小小红包一份哦!小编微信:Mayyy530
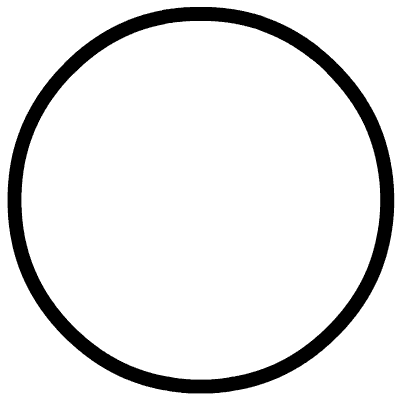
评论