使用Python+OpenCV统计每日用电量(附代码演练)
AI算法与图像处理
共 10288字,需浏览 21分钟
·
2021-03-21 20:25
点击下面卡片关注“AI算法与图像处理”,选择加"星标"或“置顶”
重磅干货,第一时间送达
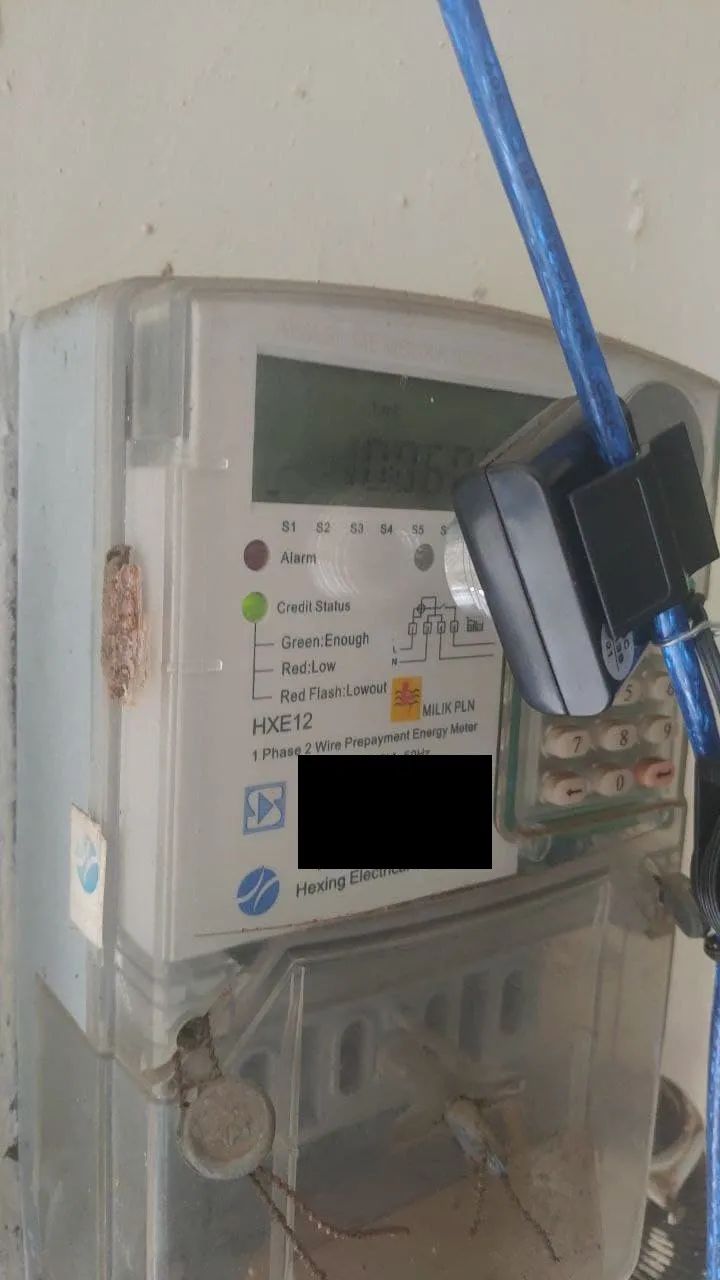
让我们编码!
kwh_pln_sensor.py
from sqlalchemy import create_engine
import cv2
import numpy as np
import pandas as pd
import datetime
import time
sqlalchemy是连接数据库(对象关系映射器)的助手,在本例中为PostgreSQL cv2(开源的计算机视觉库,或称为OpenCV):这个python库可以帮助从相机中过滤出像素颜色,用于过滤红色 pandas使创建矩阵列和行以将数据保存到数据库变得更容易 datetime和time,管理日期和时间格式的库
pip install opencv-python
pip install sqlalchemy
pip install pandas
conn =
create_engine("postgresql+psycopg2://root:12345678@localhost:5432/plnstats").connect()
cap = cv2.VideoCapture(0)
(ret, frame) = cap.read()
while True:
(ret, frame) = cap.read()
size = frame.size
image = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
将原始帧转换为RGB颜色,image= cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
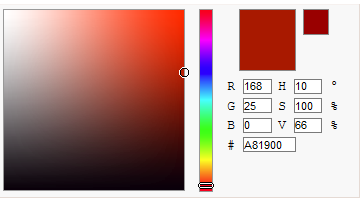
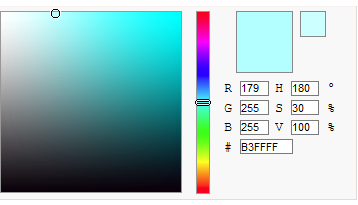
lower = np.array([168, 25, 0])
upper = np.array([179, 255, 255])
mask = cv2.inRange(image, lower, upper)
no_red = cv2.countNonZero(mask)
frac_red = np.divide(float(no_red), size)
percent_red = np.multiply((float(frac_red)), 100)
if (percent_red >= 10.0):
data_capture = {
'color_percentage': percent_red,
'created_on': datetime.datetime.now()
}
df = pd.DataFrame(columns=['color_percentage','created_on'],data=data_capture,index=[0])
df.to_sql('home_pln_kwh_sensor', schema='public', con=conn, if_exists='append',index=False)
from sqlalchemy import create_engine
import cv2
import numpy as np
import pandas as pd
import datetime
import time
conn = create_engine("postgresql+psycopg2://root:12345678@localhost:5432/plnstats").connect()
cap = cv2.VideoCapture(0)
(ret, frame) = cap.read()
capture_status = True
while capture_status:
(ret, frame) = cap.read()
size = frame.size
image = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
lower = np.array([168, 25, 0])
upper = np.array([179, 255, 255])
mask = cv2.inRange(image, lower, upper)
no_red = cv2.countNonZero(mask)
frac_red = np.divide(float(no_red), size)
percent_red = np.multiply((float(frac_red)), 100)
print(percent_red)
if (percent_red >= 10.0): #estimated from the red colour percentage impulse LED lamp
data_capture = {
'color_percentage': percent_red,
'created_on': datetime.datetime.now()
}
df = pd.DataFrame(columns=['color_percentage','created_on'],data=data_capture,index=[0])
df.to_sql('home_pln_kwh_sensor', schema='public', con=conn, if_exists='append',index=False)
cap.release()
cv2.destroyAllWindows()
kwh_pln_calculate.py
import pandas as pd
from datetime import datetime,timedelta
from sqlalchemy import create_engine
IMPULSE_KWH = 1000
RUPIAH_PER_KWH = 1444.70
now_date = datetime.now().strftime('%Y-%m-%d')
yesterday_date = datetime.now() - timedelta(days=1)
yesterday_date = yesterday_date.strftime('%Y-%m-%d')
conn = create_engine("postgresql+psycopg2://root:12345678@localhost:5432/plnstats").connect()
df = pd.read_sql(sql='SELECT color_percentage, created_on from public.home_pln_kwh_sensor where created_on >= \'{yesterday} 00:00:00\' and created_on < \'{currdate} 00:00:00\''.format(yesterday=yesterday_date,currdate=now_date),con=conn)
df['created_on'] = pd.to_datetime(df.created_on)
df['created_on'] = df['created_on'].dt.strftime('%Y-%m-%d %H:%M:%S')
df = df.drop_duplicates(subset=['created_on'])
df['created_on'] = pd.to_datetime(df.created_on)
df['hour'] = df['created_on'].dt.strftime('%H')
df['day'] = df['created_on'].dt.strftime('%Y-%m-%d')
list_day = list(df['day'].unique())
df_summary = pd.DataFrame(columns=['day','hour','sum_impulse','charges_amount'])
for dday in list_day:
list_hour = list(df[df['day'] == str(dday)]['hour'].unique())
for y in list_hour:
wSumKWH = df[(df['day'] == str(dday)) & (df['hour'] == str(y))]
df_summary = df_summary.append({'hour':y,'day':dday,'sum_impulse':wSumKWH.groupby('hour').count()['day'][0],'charges_amount':(wSumKWH.groupby('hour').count()['day'][0])*RUPIAH_PER_KWH/IMPULSE_KWH},ignore_index=True)
df_summary.to_sql('home_pln_kwh_hour', schema='public', con=conn, if_exists='append', index=False)
credit_summary = df_summary[df_summary['day'] == yesterday_date].groupby('day').sum()
credit_summary.to_sql('home_pln_kwh_summary', schema='public', con=conn, if_exists='append', index=True)
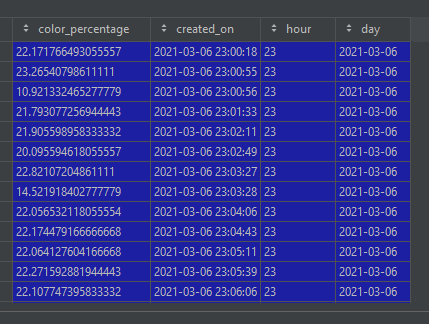
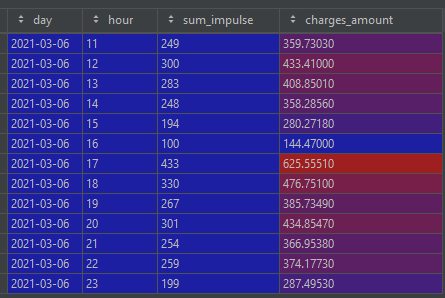
import pandas as pd
from datetime import datetime,timedelta
from sqlalchemy import create_engine
IMPULSE_KWH = 1000 #depends on prepaid meter
RUPIAH_PER_KWH = 1444.70 # R-1/TR 1.301 – 2.200 VA
def run_cron():
now_date = datetime.now().strftime('%Y-%m-%d')
yesterday_date = datetime.now() - timedelta(days=1)
yesterday_date = yesterday_date.strftime('%Y-%m-%d')
conn = create_engine("postgresql+psycopg2://root:12345678@localhost:5432/plnstats").connect()
df = pd.read_sql(sql='SELECT color_percentage, created_on from public.home_pln_kwh_sensor where created_on >= \'{yesterday} 00:00:00\' and created_on < \'{currdate} 00:00:00\''.format(yesterday=yesterday_date,currdate=now_date),con=conn)
df['created_on'] = pd.to_datetime(df.created_on)
df['created_on'] = df['created_on'].dt.strftime('%Y-%m-%d %H:%M:%S')
df = df.drop_duplicates(subset=['created_on'])
df['created_on'] = pd.to_datetime(df.created_on)
df['hour'] = df['created_on'].dt.strftime('%H')
df['day'] = df['created_on'].dt.strftime('%Y-%m-%d')
list_day = list(df['day'].unique())
df_summary = pd.DataFrame(columns=['day','hour','sum_impulse','charges_amount'])
#DETAIL PER HOUR
for dday in list_day:
list_hour = list(df[df['day'] == str(dday)]['hour'].unique())
for y in list_hour:
wSumKWH = df[(df['day'] == str(dday)) & (df['hour'] == str(y))]
df_summary = df_summary.append({'hour':y,'day':dday,'sum_impulse':wSumKWH.groupby('hour').count()['day'][0],'charges_amount':(wSumKWH.groupby('hour').count()['day'][0])*RUPIAH_PER_KWH/IMPULSE_KWH},ignore_index=True)
df_summary.to_sql('home_pln_kwh_hour', schema='public', con=conn, if_exists='append', index=False)
credit_summary = df_summary[df_summary['day'] == yesterday_date].groupby('day').sum()
credit_summary.to_sql('home_pln_kwh_summary', schema='public', con=conn, if_exists='append', index=True)
if __name__ == '__main__':
run_cron()
个人微信(如果没有备注不拉群!) 请注明:地区+学校/企业+研究方向+昵称
下载1:何恺明顶会分享
在「AI算法与图像处理」公众号后台回复:何恺明,即可下载。总共有6份PDF,涉及 ResNet、Mask RCNN等经典工作的总结分析
下载2:终身受益的编程指南:Google编程风格指南
在「AI算法与图像处理」公众号后台回复:c++,即可下载。历经十年考验,最权威的编程规范!
下载3 CVPR2021 在「AI算法与图像处理」公众号后台回复:CVPR,即可下载1467篇CVPR 2020论文 和 CVPR 2021 最新论文
点亮 ,告诉大家你也在看
评论