如何更容易上手 TypeScript 类型编程?
点击上方“前端简报”,选择“设为星标”
第一时间关注技术干货!
1. Why
在介绍什么叫 TypeScript 类型编程和为什么需要学习 TypeScript 类型编程之前,我们先看一个例子,这里例子里包含一个 promisify 的函数,这个函数用于将 NodeJS 中 callback style 的函数转换成 promise style 的函数。
import * as fs from "fs";
function promisify(fn) {
return function(...args) {
return new Promise((resolve, reject) => {
fn(...args, (err, data) => {
if(err) {
return reject(err);
}
resolve(data);
});
});
}
}
(async () => {
let file = await promisify(fs.readFile)("./xxx.json");
})();
如果我们直接套用上述的代码,那么 file 的类型和 promisify(fs.readFile)(...)
中 (...)
的类型也会丢失,也就是我们有两个目标:
我们需要知道
promisify(fs.readFile)(...)
这里能够接受的类型。我们需要知道
let file = await ...
这里 file 的类型。
这个问题的答案在实战演练环节会结合本文的内容给出答案,如果你觉得这个问题简单得很,那么恭喜你,你已经具备本文将要介绍的大部分知识点。如何让类似于 promisify这样的函数保留类型信息是“体操”或者我称之为类型编程的意义所在。
2. 前言 (Preface)
最近在国内的前端圈流行一个名词“TS 体操”,简称为“TC”,体操这个词是从 Haskell 社区来的,本意就是高难度动作,关于“体操”能够实现到底多高难度的动作,可以参照下面这篇文章。
https://www.zhihu.com/question/418792736/answer/1448121319[1]
3. 建模 (Modeling)
4. 语法分类 (Grammar Classification)
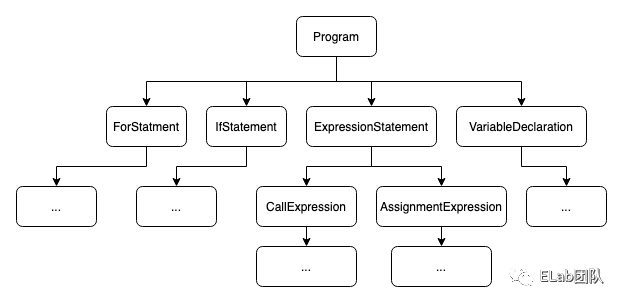
4.1 基本类型 (Basic Types)
Boolean[4] Number[5] String[6] Array[7] Tuple[8] (TypeScript 独有) Enum[9] (TypeScript 独有) Unknown[10] (TypeScript 独有) Any[11] (TypeScript 独有) Void[12] (TypeScript 独有) Null and Undefined[13] Never[14] (TypeScript 独有) Object[15]
type A = {
attrA: string,
attrB: number,
attrA: true, // Boolean 的枚举
...
}
4.2 函数 (Function)
类比 let func = (argA, argB, ...) => expression;
// 函数定义
type B<T> = T & {
attrB: "anthor value"
}
// 变量
class CCC {
...
}
type DDD = {
...
}
// 函数调用
type AnotherType = B<CCC>;
type YetAnotherType = B<DDD>;
<T>
就相当于函数括弧和参数列表,=
后面的就相当于函数定义。或者按照这个思路你可以开始沉淀很多工具类 TC 函数了,例如// 将所有属性变成可选的
type Optional<T> = {
[key in keyof T]?: T[key];
}
// 将某些属性变成必选的
type MyRequired<T, K extends keyof T> = T &
{
[key in K]-?: T[key];
};
// 例如我们有个实体
type App = {
_id?: string;
appId: string;
name: string;
description: string;
ownerList: string[];
createdAt?: number;
updatedAt?: number;
};
// 我们在更新这个对象/类型的时候,有些 key 是必填的,有些 key 是选填的,这个时候就可以这样子生成我们需要的类型
type AppUpdatePayload = MyRequired<Optional<App>, '_id'>
<K extends keyof T>
这样的语法来表达。TypeScript 函数的缺陷 (Defect)
高版本才能支持递归
函数不能作为参数
function map(s, mapper) { return s.map(mapper) }
map([1, 2, 3], (t) => s);
type Map<T, Mapper> = {
[k in keyof T]: Mapper<T[k]>; // 语法报错
}
支持闭包,但是没有办法修改闭包中的值
type ClosureValue = string;
type Map<T> = {
[k in keyof T]: ClosureValue; // 笔者没有找到语法能够修改 ClosureValue
}
type ClosureValue = string;
type Map<T> = {
[k in keyof T]: ClosureValue & T[k]; // 笔者没有找到语法能够修改 ClosureValue
}
4.3 语句 (Statements)
变量声明语句 (Variable Declaration)
类比:let a = Expression;
type ToDeclareType = Expresion
这样子的变量名加表达式的语法来实现,表达式有很多种类,我们接下来会详细到介绍到,type ToDeclareType<T> = T extends (args: any) => PromiseLike<infer R> ? R : never; // 条件表达式/带三元运算符的条件表达式
type ToDeclareType = Omit<App>; // 函数调用表达式
type ToDeclareType<T>= { // 循环表达式
[key in keyof T]: Omit<T[key], '_id'>
}
4.4 表达式 (Expressions)
带三元运算符的条件表达式 (IfExpression with ternary operator)
类比:a == b ? 'hello' : 'world';
Condition ? ExpressionIfTrue : ExpressionIfFalse
这样的形式,在 TypeScript 中则可以用以下的语法来表示:type TypeOfWhatPromiseReturn<T> = T extends (args: any) => PromiseLike<infer R> ? R : never;
T extends (args: any) => PromiseLike<infer R>
就相当条件判断,R : never
就相当于为真时的表达式和为假时的表达式。async function hello(name: string): Promise<string> {
return Promise.resolve(name);
}
// type CCC: string = ReturnType<typeof hello>; doesn't work
type MyReturnType<T extends (...args) => any> = T extends (
...args
) => PromiseLike<infer R>
? R
: ReturnType<T>;
type CCC: string = MyReturnType<typeof hello>; // it works
函数调用/定义表达式 (CallExpression)
类比:call(a, b, c);
循环相关 (Loop Related)(Object.keys、Array.map等)
类比:for (let k in b) { ... }
循环实现思路 (Details Explained )
注意:递归只有在 TS 4.1.0 才支持
type IntSeq<N, S extends any[] = []> =
S["length"] extends N ? S :
IntSeq<N, [...S, S["length"]]>
对对象进行遍历 (Loop Object)
type AnyType = {
[key: string]: any;
};
type OptionalString<T> = {
[key in keyof T]?: string;
};
type CCC = OptionalString<AnyType>;
对数组(Tuple)进行遍历 (Loop Array/Tuple)
map
类比:Array.map
const a = ['123', 1, {}];
type B = typeof a;
type Map<T> = {
[k in keyof T]: T[k] extends (...args) => any ? 0 : 1;
};
type C = Map<B>;
type D = C[0];
reduce
类比:Array.reduce
const a = ['123', 1, {}];
type B = typeof a;
type Reduce<T extends any[]> = T[number] extends (...arg: any[]) => any ? 1 : 0;
type C = Reduce<B>;
4.5 成员表达式 (Member Expression)
a.b.c
这样的成员表达式主要是因为我们知道了某个对象/变量的结构,然后想拿到其中某部分的值,在 TypeScript 中有个比较通用的方法,就是用 infer 语法,例如我们想拿到函数的某个参数就可以这么做:function hello(a: any, b: string) {
return b;
}
type getSecondParameter<T> = T extends (a: any, b: infer U) => any ? U : never;
type P = getSecondParameter<typeof hello>;
T extends (a: any, b: infer U) => any
就是在表示结构,并拿其中某个部分。type A = {
a: string;
b: string;
};
type B = [string, string, boolean];
type C = A['a'];
type D = B[number];
type E = B[0];
// eslint-disable-next-line prettier/prettier
type Last<T extends any[]> = T extends [...infer _, infer L] ? L : never;
type F = Last<B>;
4.6 常见数据结构和操作 (Common Datastructures and Operations)
Set
Add
type S = '1' | 2 | a;
S = S | 3;
Remove
type S = '1' | 2 | a;
S = Exclude<S, '1'>;
Has
type S = '1' | 2 | a;
type isInSet = 1 extends S ? true : false;
Intersection
type SA = '1' | 2;
type SB = 2 | 3;
type interset = Extract<SA, SB>;
Diff
type SA = '1' | 2;
type SB = 2 | 3;
type diff = Exclude<SA, SB>;
Symmetric Diff
type SA = '1' | 2;
type SB = 2 | 3;
type sdiff = Exclude<SA, SB> | Exclude<SB, SA>;
ToIntersectionType
type A = {
a: string;
b: string;
};
type B = {
b: string;
c: string;
};
type ToIntersectionType<U> = (
U extends any ? (arg: U) => any : never
) extends (arg: infer I) => void
? I
: never;
type D = ToIntersectionType <A | B>;
ToArray
注意:递归只有在 TS 4.1.0 才支持
type Input = 1 | 2;
type UnionToIntersection<U> = (
U extends any ? (arg: U) => any : never
) extends (arg: infer I) => void
? I
: never;
type ToArray<T> = UnionToIntersection<(T extends any ? (t: T) => T : never)> extends (_: any) => infer W
? [...ToArray<Exclude<T, W>>, W]
: [];
type Output = ToArray<Input>;
type C = ((arg: any) => true) & ((arg: any) => false);
type D = C extends (arg: any) => infer R ? R : never; // false;
Size
type Input = 1 | 2;
type Size = ToArray<Input>['length'];
Map/Object
Merge/Object.assign
type C = A & B;
Intersection
interface A {
a: string;
b: string;
c: string;
}
interface B {
b: string;
c: number;
d: boolean;
}
type Intersection<A, B> = {
[KA in Extract<keyof A, keyof B>]: A[KA] | B[KA];
};
type AandB = Intersection<A, B>;
Filter
type Input = { foo: number; bar?: string };
type FilteredKeys<T> = {
[P in keyof T]: T[P] extends number ? P : never;
}[keyof T];
type Filter<T> = {
[key in FilteredKeys<T>]: T[key];
};
type Output = Filter<Input>;
Array
成员访问
type B = [string, string, boolean];
type D = B[number];
type E = B[0];
// eslint-disable-next-line prettier/prettier
type Last<T extends any[]> = T extends [...infer _, infer L] ? L : never;
type F = Last<B>;
type G = B['length'];
Append
type Append<T extends any[], V> = [...T, V];
Pop
type Pop<T extends any[]> = T extends [...infer I, infer _] ? I : never
Dequeue
type Dequeue<T extends any[]> = T extends [infer _, ...infer I] ? I : never
Prepend
type Prepend<T extends any[], V> = [V, ...T];
Concat
type Concat<T extends any[], V extends any[] > = [...T, ...V];
Filter
注意:递归只有在 TS 4.1.0 才支持
type Filter<T extends any[]> = T extends [infer V, ...infer R]
? V extends number
? [V, ...Filter<R>]
: Filter<R>
: [];
type Input = [1, 2, string];
type Output = Filter<Input>;
Slice
注意:递归只有在 TS 4.1.0 才支持 注意:为了实现简单,这里 Slice 的用法和 Array.slice 用法不一样:N 表示剩余元素的个数。
type Input = [string, string, boolean];
type Slice<N extends number, T extends any[]> = T['length'] extends N
? T
: T extends [infer _, ...infer U]
? Slice<N, U>
: never;
type Out = Slice<2, Input>;
Array.slice(s)
这种效果,实现 Array.slice(s, e)
涉及减法,比较麻烦,暂不在这里展开了。4.7 运算符 (Operators)
注意:运算符的实现涉及递归,递归只有在 TS 4.1.0 才支持
注意:下面的运算符只能适用于整型
注意:原理依赖于递归、效率较低
基本原理 (Details Explained)
type IntSeq<N, S extends any[] = []> =
S["length"] extends N ? S :
IntSeq<N, [...S, S["length"]]>;
===
type IfEquals<X, Y, A = X, B = never> = (<T>() => T extends X ? 1 : 2) extends <
T
>() => T extends Y ? 1 : 2
? A
: B;
+
type NumericPlus<A extends Numeric, B extends Numeric> = [...IntSeq<A>, ...IntSeq<B>]["length"];
-
注意:减法结果不支持负数 ...
type NumericMinus<A extends Numeric, B extends Numeric> = _NumericMinus<B, A, []>;
type ToNumeric<T extends number> = T extends Numeric ? T : never;
type _NumericMinus<A extends Numeric, B extends Numeric, M extends any[]> = NumericPlus<A, ToNumeric<M["length"]>> extends B ? M["length"] : _NumericMinus<A, B, [...M, 0]>;
4.8 其他 (MISC)
inferface
inteface A extends B {
attrA: string
}
Utility Types
5. 实战演练 (Excercise)
Promisify
import * as fs from "fs";
function promisify(fn) {
return function(...args: XXXX) {
return new Promise<XXXX>((resolve, reject) => {
fn(...args, (err, data) => {
if(err) {
return reject(err);
}
resolve(data);
});
});
}
}
(async () => {
let file = await promisify(fs.readFile)("./xxx.json");
})();
我们需要知道 promisify(fs.readFile)(...)
这里能够接受的类型。我们需要 let file = await ...
这里 file 的类型。
答案
import * as fs from "fs";
// 基于数据的基本操作 Last 和 Pop
type Last<T extends any[]> = T extends [...infer _, infer L] ? L : never;
type Pop<T extends any[]> = T extends [...infer I, infer _] ? I : never;
// 对数组进行操作
type GetParametersType<T extends (...args: any) => any> = Pop<Parameters<T>>;
type GetCallbackType<T extends (...args: any) => any> = Last<Parameters<T>>;
// 类似于成员变量取值
type GetCallbackReturnType<T extends (...args: any) => any> = GetCallbackType<T> extends (err: Error, data: infer R) => void ? R : any;
function promisify<T extends (...args: any) => any>(fn: T) {
return function(...args: GetParametersType<T>) {
return new Promise<GetCallbackReturnType<T>>((resolve, reject) => {
fn(...args, (err, data) => {
if(err) {
return reject(err);
}
resolve(data);
});
});
}
}
(async () => {
let file = await promisify(fs.readFile)("./xxx.json");
})();
MyReturnType[19]
const fn = (v: boolean) => {
if (v) return 1;
else return 2;
};
type MyReturnType<F> = F extends (...args) => infer R ? R : never;
type a = MyReturnType<typeof fn>;
Readonly 2[20]
interface Todo {
title: string;
description: string;
completed: boolean;
}
type MyReadonly2<T, KEYS extends keyof T> = T &
{
readonly [k in KEYS]: T[k];
};
const todo: MyReadonly2<Todo, 'title' | 'description'> = {
title: 'Hey',
description: 'foobar',
completed: false,
};
todo.title = 'Hello'; // Error: cannot reassign a readonly property
todo.description = 'barFoo'; // Error: cannot reassign a readonly property
todo.completed = true; // O
Type Lookup[21]
interface Cat {
type: 'cat';
breeds: 'Abyssinian' | 'Shorthair' | 'Curl' | 'Bengal';
}
interface Dog {
type: 'dog';
breeds: 'Hound' | 'Brittany' | 'Bulldog' | 'Boxer';
color: 'brown' | 'white' | 'black';
}
type LookUp<T, K extends string> = T extends { type: string }
? T['type'] extends K
? T
: never
: never;
type MyDogType = LookUp<Cat | Dog, 'dog'>; // expected to be `Dog`
Get Required[22]
type GetRequiredKeys<T> = {
[key in keyof T]-?: {} extends Pick<T, key> ? never : key;
}[keyof T];
type GetRequired<T> = {
[key in GetRequiredKeys<T>]: T[key];
};
type I = GetRequired<{ foo: number; bar?: string }>; // expected to be { foo: number }
6. 想法 (Thoughts)
沉淀类型编程库 (Supplementary Utility Types)
https://github.com/piotrwitek/utility-types
https://github.com/sindresorhus/type-fest
直接用 JS 做类型编程 (Doing Type Computing in Plain TS)
type Test = {
a: string
}
typecomp function Map(T, mapper) {
for (let key of Object.keys(T)) {
T[key] = mapper(T[key]);
}
}
typecomp AnotherType = Map(Test, typecomp (T) => {
if (T extends 'hello') {
return number;
} else {
return string;
}
});
7. Reference
https://github.com/type-challenges/type-challenges https://www.zhihu.com/question/418792736/answer/1448121319 https://github.com/piotrwitek/utility-types#requiredkeyst
参考资料
[1] https://www.zhihu.com/question/418792736/answer/1448121319
[2] 有趣的 brain teaser: https://github.com/type-challenges/type-challenges
[3] AST(抽象语法树)的角度来看: https://github.com/babel/babel/blob/main/packages/babel-types/src/definitions/core.js
[4] Boolean: https://www.typescriptlang.org/docs/handbook/basic-types.html#boolean
[5] Number: https://www.typescriptlang.org/docs/handbook/basic-types.html#number
[6] String: https://www.typescriptlang.org/docs/handbook/basic-types.html#string
[7] Array: https://www.typescriptlang.org/docs/handbook/basic-types.html#array
[8] Tuple: https://www.typescriptlang.org/docs/handbook/basic-types.html#tuple
[9] Enum: https://www.typescriptlang.org/docs/handbook/basic-types.html#enum
[10] Unknown: https://www.typescriptlang.org/docs/handbook/basic-types.html#unknown
[11] Any: https://www.typescriptlang.org/docs/handbook/basic-types.html#any
[12] Void: https://www.typescriptlang.org/docs/handbook/basic-types.html#void
[13] Null and Undefined: https://www.typescriptlang.org/docs/handbook/basic-types.html#null-and-undefined
[14] Never: https://www.typescriptlang.org/docs/handbook/basic-types.html#never
[15] Object: https://www.typescriptlang.org/docs/handbook/basic-types.html#object
[16] Declaration Merging: https://www.typescriptlang.org/docs/handbook/declaration-merging.html
[17] 这个链接: https://www.typescriptlang.org/docs/handbook/utility-types.html
[18] https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/types/util.promisify/implementation.d.ts
[19] https://github.com/type-challenges/type-challenges/blob/master/questions/2-medium-return-type/README.md
[20] https://github.com/type-challenges/type-challenges/blob/master/questions/8-medium-readonly-2/README.md
[21] https://github.com/type-challenges/type-challenges/blob/master/questions/62-medium-type-lookup/README.md
[22] https://github.com/type-challenges/type-challenges/blob/master/questions/57-hard-get-required/README.md