社区精选|一文带你读懂 javascript 中的箭头函数
SegmentFault
共 9514字,需浏览 20分钟
·
2023-07-29 00:15
今天小编为大家带来的是社区作者 风如也 的文章,让我们一起来学习 Javascript 中的箭头函数
箭头函数
(param1, param2, …, paramN) => { statements }
(param1, param2, …, paramN) => expression
//相当于:(param1, param2, …, paramN) =>{ return expression; }
// 当只有一个参数时,圆括号是可选的:
(singleParam) => { statements }
singleParam => { statements }
// 没有参数的函数应该写成一对圆括号。
() => { statements }
//加括号的函数体返回对象字面量表达式:
params => ({foo: bar})
//支持剩余参数和默认参数
(param1, param2, ...rest) => { statements }
(param1 = defaultValue1, param2, …, paramN = defaultValueN) => {
statements }
//同样支持参数列表解构
let f = ([a, b] = [1, 2], {x: c} = {x: a + b}) => a + b + c;
f(); // 6
没有单独的this
function foo() {
setTimeout(() => {
console.log('id:', this.id);
}, 100);
}
var id = 21;
foo.call({ id: 42 }); // id: 42
通过 call 或 apply 或 bind 调用
var adder = {
base : 1,
add: function(a) {
var f = v => v + this.base;
return f(a);
},
addAnotherThis: function(a) {
var f = v => v + this.base;
var b = {
base : 2
};
// return f.call(b, a);
// return f.apply(b, [a]);
return f.bind(b, a)();
}
};
console.log(adder.add(1)); // 输出 2
console.log(adder.addAnotherThis(1)); // 输出 2
不绑定 arguments
var arguments = [1, 2, 3];
var fn = () => arguments[0];
// arguments 只是引用了封闭作用域内的 arguments
fn(); // 1
function getArgs(n) {
// 隐式绑定 getArgs 函数的 arguments 对象。arguments[0] 是 n
// 即传给 getArgs 函数的第一个参数
var f = () => arguments[0] + n;
return f();
}
getArgs(1); // 2
getArgs(2); // 4
getArgs(3); // 6
getArgs(3,2);//6
function getArgs(n) {
var f = (...args) => args[0];
return f();
}
getArgs(1); // 1
getArgs(2); // 2
const numbers = (...nums) => nums;
使用箭头函数作为方法
var obj = {
a: 10,
b: () => console.log(this.a, this),
c: function() {
console.log(this.a, this)
}
}
obj.b();
// undefined, Window{...}
obj.c();
// 10, Object {...}
let obj = {
a: 10
};
Object.defineProperty(obj, "b", {
get: () => {
console.log(this.a, typeof this.a, this);
return this.a + 10;
// this 代表全局对象 'Window', 因此 'this.a' 返回 'undefined'
}
});
不能使用 new 操作符
let Apple = () => {}
let apple = new Apple() // Uncaught TypeError: Apple is not a constructor
不能使用 prototype 属性
let far = function() {}
console.log(far.prototype) // {constructor: ƒ}
let Apple = () => {}
console.log(Apple.prototype) // undefined
不能用作函数生成器
返回对象字面量
let apple = () => ({ height: 100 })
换行
let apple = ()
=> ({ height: 100 }) // Uncaught SyntaxError: Unexpected token '=>'
let apple = () =>
({ height: 100 })
let apple = (
s, a, b
) =>
({ height: 100 })
let apple = () => {
// ...
}
箭头函数不适用场合
const obj = {
a: 1,
fn: () => {
this.a++
}
}
console.log(obj.fn()) // undefined
var button = document.getElementById('press');
button.addEventListener('click', () => {
this.classList.toggle('on');
});
往期推荐
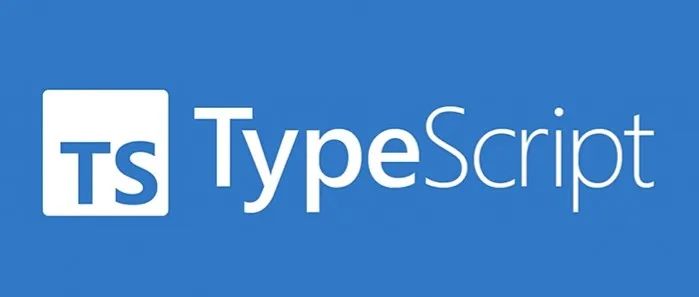
社区精选|TypeScript 玩转类型操作
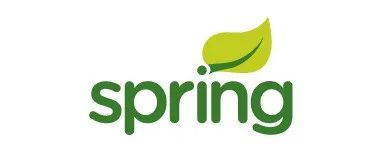
社区精选Spring5 中更优雅的第三方 Bean 注入
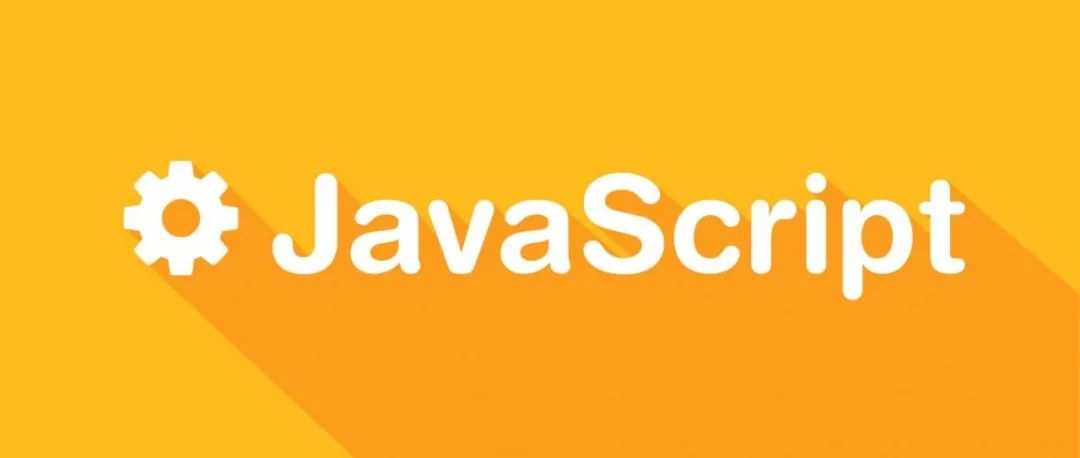
社区精选|JavaScript 内存泄漏
评论