LeetCode刷题实战87: 扰乱字符串
共 3065字,需浏览 7分钟
·
2020-11-07 02:17
算法的重要性,我就不多说了吧,想去大厂,就必须要经过基础知识和业务逻辑面试+算法面试。所以,为了提高大家的算法能力,这个公众号后续每天带大家做一道算法题,题目就从LeetCode上面选 !
今天和大家聊的问题叫做 扰乱字符串,我们先来看题面:
https://leetcode-cn.com/problems/scramble-string/
We can scramble a string s to get a string t using the following algorithm:
If the length of the string is 1, stop.
If the length of the string is > 1, do the following:
Split the string into two non-empty substrings at a random index, i.e., if the string is s, divide it to x and y where s = x + y.
Randomly decide to swap the two substrings or to keep them in the same order. i.e., after this step, s may become s = x + y or s = y + x.
Apply step 1 recursively on each of the two substrings x and y.
Given two strings s1 and s2 of the same length, return true if s2 is a scrambled string of s1, otherwise, return false.
题意
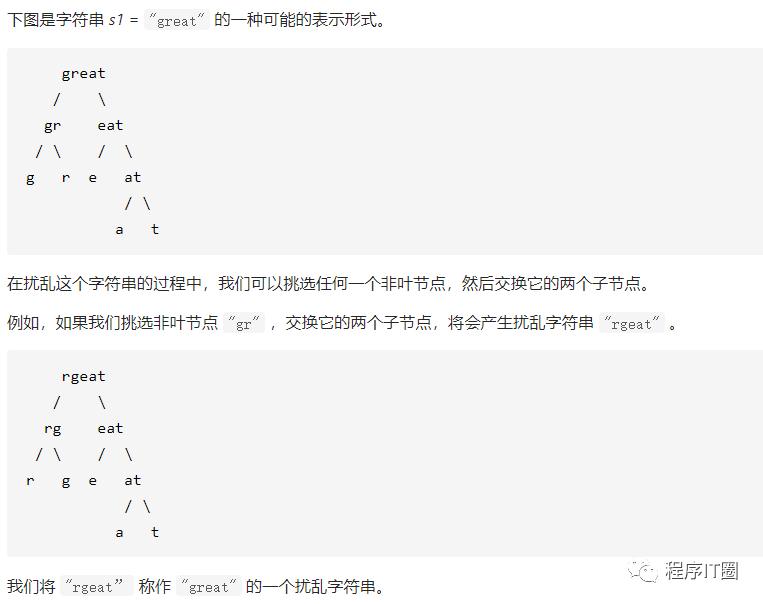
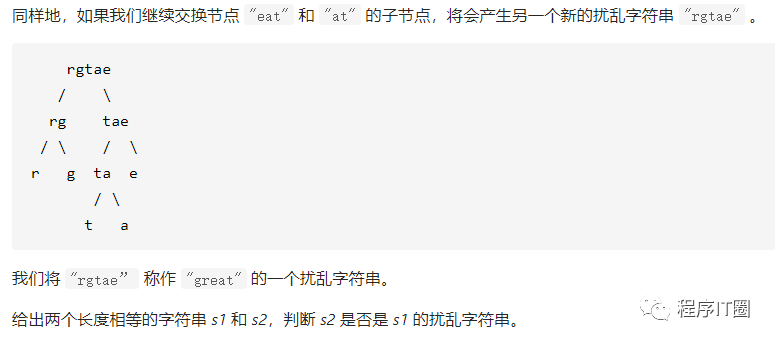
示例 1:
输入: s1 = "great", s2 = "rgeat"
输出: true
示例 2:
输入: s1 = "abcde", s2 = "caebd"
输出: false
解题
题解
class Solution:
def isScramble(self, s1: str, s2: str) -> bool:
from collections import Counter
def determine(s1, s2):
# 如果s1和s2构成的字符不同,那么直接排除
c1 = Counter(list(s1))
c2 = Counter(list(s2))
return c1 == c2
def dfs(s1, s2):
# 如果要判断的s1和s2相等,返回True
if s1 == s2:
return True
if not determine(s1, s2):
return False
n = len(s1)
# 枚举拆分的位置将字符串拆分成两个部分
for i in range(1, n):
if dfs(s1[:i], s2[:i]) and dfs(s1[i:], s2[i:]) or dfs(s1[:i], s2[n-i:]) and dfs(s1[i:], s2[:n-i]):
return True
return False
if len(s1) != len(s2):
return False
if len(s1) == 0:
return True
return dfs(s1, s2)
总结
上期推文: