如何用 JavaScript 来解析 URL
web前端开发
共 4168字,需浏览 9分钟
·
2020-09-20 05:09
https://dmitripavlutin.com/parse-url-JavaScript
1、URL 结构
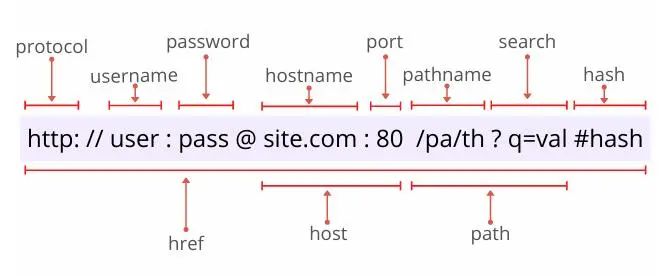
2、URL() 构造函数
const url = new URL(relativeOrAbsolute [, absoluteBase]);
const url = new URL('http://example.com/path/index.html');
url.href; // => 'http://example.com/path/index.html'
const url = new URL('/path/index.html', 'http://example.com');
url.href; // => 'http://example.com/path/index.html'
interface URL {
href: USVString;
protocol: USVString;
username: USVString;
password: USVString;
host: USVString;
hostname: USVString;
port: USVString;
pathname: USVString;
search: USVString;
hash: USVString;
readonly origin: USVString;
readonly searchParams: URLSearchParams;
tojsON(): USVString;
}
3、Query 字符串
const url = new URL(
'http://example.com/path/index.html?message=hello&who=world'
);
url.search; // => '?message=hello&who=world'
const url1 = new URL('http://example.com/path/index.html');
const url2 = new URL('http://example.com/path/index.html?');
url1.search; // => ''
url2.search; // => ''
3.1 、解析 query 字符串
const url = new URL(
'http://example.com/path/index.html?message=hello&who=world'
);
url.searchParams.get('message'); // => 'hello'
url.searchParams.get('missing'); // => null
4、hostname
const url = new URL('http://example.com/path/index.html');
url.hostname; // => 'example.com'
5、pathname
const url = new URL('http://example.com/path/index.html?param=value');
url.pathname; // => '/path/index.html'
const url = new URL('http://example.com/');
url.pathname; // => '/'
6、hash
const url = new URL('http://example.com/path/index.html#bottom');
url.hash; // => '#bottom'
const url = new URL('http://example.com/path/index.html');
url.hash; // => ''
7、URL 校验
try {
const url = new URL('http ://example.com');
} catch (error) {
error; // => TypeError, "Failed to construct URL: Invalid URL"
}
8、修改 URL
const url = new URL('http://red.com/path/index.html');
url.href; // => 'http://red.com/path/index.html'
url.hostname = 'blue.io';
url.href; // => 'http://blue.io/path/index.html'
9、总结
- url.search 获取原生的 query 字符串
- url.searchParams 通过 URLSearchParams 的实例去获取具体的 query 参数
- url.hostname获取 hostname
- url.pathname 获取 pathname
- url.hash 获取 hash 值

评论