LeetCode刷题实战445:两数相加 II
共 2099字,需浏览 5分钟
·
2021-11-26 19:46
You are given two non-empty linked lists representing two non-negative integers. The most significant digit comes first and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
示例
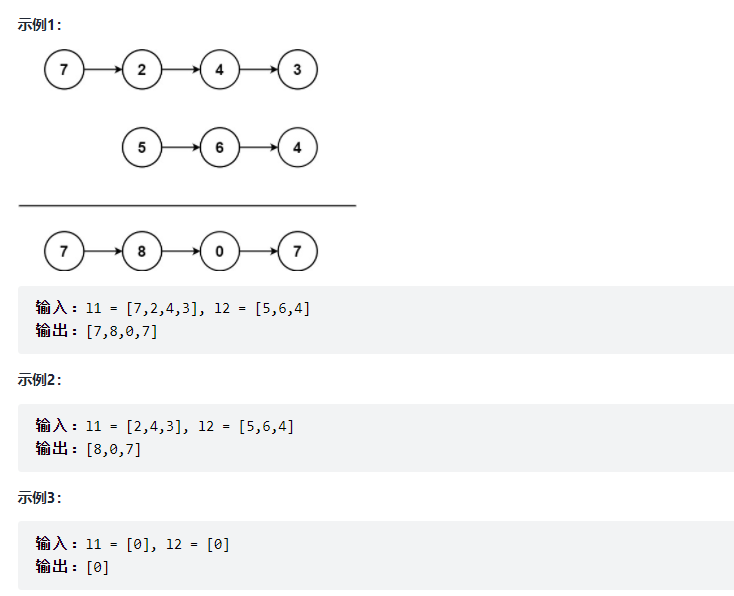
解题
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def addTwoNumbers(self, l1: ListNode, l2: ListNode) -> ListNode:
# 定义两个栈用于存储链表中的节点值
stack1, stack2 = [], []
# 最后的结果链表
ans = None
# 进位
carry = 0
while l1:
stack1.append(l1.val)
l1 = l1.next
while l2:
stack2.append(l2.val)
l2 = l2.next
while stack1 or stack2 or carry != 0:
a = 0 if not stack1 else stack1.pop()
b = 0 if not stack2 else stack2.pop()
num = a + b + carry
# res是余数,就是要生成的新节点的值
carry, res = divmod(num, 10)
# cur_node是从低位到高位来建立新的节点,根据是出栈的值是低位先出栈来计算
cur_node = ListNode(res)
cur_node.next = ans
# ans左移
ans = cur_node
return ans