Python100经典练习题.pdf(附答案)


点击上方蓝字关注我们
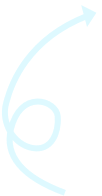
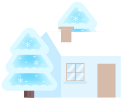
点击上方“印象python”,选择“星标”公众号
重磅干货,第一时间送达!
作者:Python芸芸
链接:https://www.jianshu.com/p/232d3798af55
1:Python有哪些特点和优点?
具有动态特性
面向对象
简明简单
开源
具有强大的社区支持
2:深拷贝和浅拷贝之间的区别是什么?
>>> import copy
>>> b=copy.deepcopy(a)
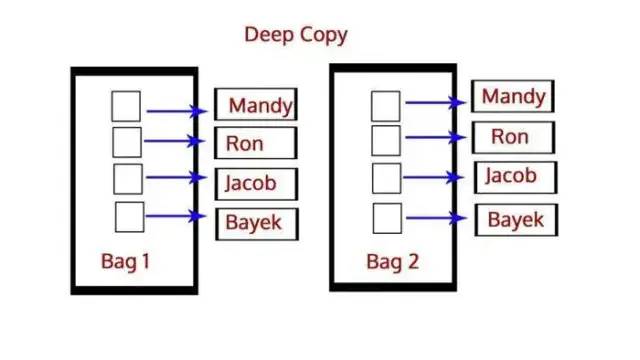
>>> b=copy.copy(a)
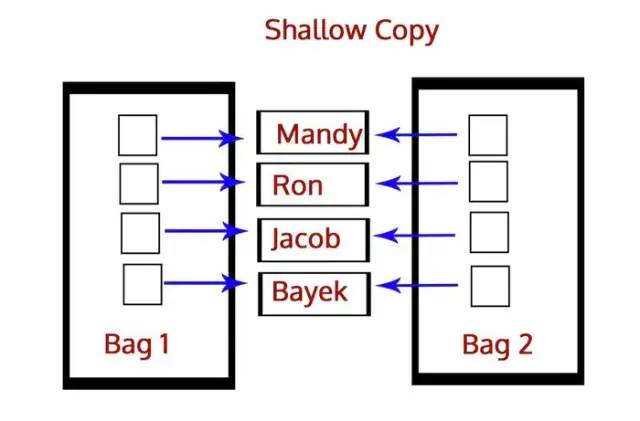
3. 列表和元组之间的区别是?
>>> mylist=[1,3,3]
>>> mylist[1]=2
>>> mytuple=(1,3,3)
>>> mytuple[1]=2
Traceback (most recent call last):
File "
", line 1, in mytuple[1]=2
TypeError: ‘tuple’ object does not support item assignment
4. 解释一下Python中的三元运算子
[on true] if [expression] else [on false]
下面是使用它的方法:
>>> a,b=2,3
>>> min=a if a
>>> min
运行结果:2
>>> print("Hi") if a
运行结果:Hi
5. 在Python中如何实现多线程?
6. 解释一下Python中的继承
单继承:一个类继承自单个基类
多继承:一个类继承自多个基类
多级继承:一个类继承自单个基类,后者则继承自另一个基类
分层继承:多个类继承自单个基类
混合继承:两种或多种类型继承的混合
7. 什么是Flask?
8. 在Python中是如何管理内存的?
9. 解释Python中的help()和dir()函数
>>> import copy
>>> help(copy.copy)
Help on function copy in module copy:
copy(x)
Shallow copy operation on arbitrary Python objects.
See the module’s __doc__ string for more info.
>>> dir(copy.copy)
[‘__annotations__’, ‘__call__’, ‘__class__’, ‘__closure__’, ‘__code__’, ‘__defaults__’, ‘__delattr__’, ‘__dict__’, ‘__dir__’, ‘__doc__’, ‘__eq__’, ‘__format__’, ‘__ge__’, ‘__get__’, ‘__getattribute__’, ‘__globals__’, ‘__gt__’, ‘__hash__’, ‘__init__’, ‘__init_subclass__’, ‘__kwdefaults__’, ‘__le__’, ‘__lt__’, ‘__module__’, ‘__name__’, ‘__ne__’, ‘__new__’, ‘__qualname__’, ‘__reduce__’, ‘__reduce_ex__’, ‘__repr__’, ‘__setattr__’, ‘__sizeof__’, ‘__str__’, ‘__subclasshook__’]
10. 当退出Python时,是否释放全部内存?
11. 什么是猴子补丁?
>>> class A:
def func(self):
print("Hi")
>>> def monkey(self):
print "Hi, monkey"
>>> m.A.func = monkey
>>> a = m.A()
>>> a.func()
Hi, Monkey
12. Python中的字典是什么?
>>> roots={25:5,16:4,9:3,4:2,1:1}
>>> type(roots)
>>> roots[9]
3
>>> roots={x**2:x for x in range(5,0,-1)}
>>> roots
{25: 5, 16: 4, 9: 3, 4: 2, 1: 1}
13. 请解释使用args和*kwargs的含义
>>> def func(*args):
for i in args:
print(i)
>>> func(3,2,1,4,7)
3
2
1
4
7
>>> def func(**kwargs):
for i in kwargs:
print(i,kwargs[i])
>>> func(a=1,b=2,c=7)
a.1
b.2c.7
14. 请写一个Python逻辑,计算一个文件中的大写字母数量
>>> import os
>>> os.chdir('C:\\Users\\lifei\\Desktop')
>>> with open('Today.txt') as today:
count=0
for i in today.read():
if i.isupper():
count+=1
print(count)
26
15. 什么是负索引?
>>> mylist=[0,1,2,3,4,5,6,7,8]
>>> mylist[-3]
6
>>> mylist[-6:-1]
[3, 4, 5, 6, 7]
16. 如何以就地操作方式打乱一个列表的元素?
>>> from random import shuffle
>>> shuffle(mylist)
>>> mylist
复制代码
[3, 4, 8, 0, 5, 7, 6, 2, 1]
17. 解释Python中的join()和split()函数
>>> ','.join('12345')
‘1,2,3,4,5’
>>> '1,2,3,4,5'.split(',')
[‘1’, ‘2’, ‘3’, ‘4’, ‘5’]
18. Python区分大小写吗?
>>> myname='Ayushi'
>>> Myname
Traceback (most recent call last):
File "
", line 1, in
Myname
NameError: name ‘Myname’ is not defined
19. Python中的标识符长度能有多长?
其余部分可以使用 A-Z/a-z/0-9
区分大小写
关键字不能作为标识符,Python中共有如下关键字:
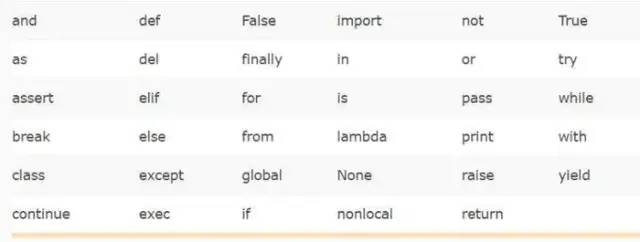
20. 怎么移除一个字符串中的前导空格?
>>> ' Ayushi '.lstrip()
‘Ayushi ’
>>> ' Ayushi '.rstrip()
‘ Ayushi’
21. 怎样将字符串转换为小写?
>>> 'AyuShi'.lower()
‘ayushi’
>>> 'AyuShi'.upper()
‘AYUSHI’
>>> 'AyuShi'.isupper()
False
>>> 'AYUSHI'.isupper()
True
>>> 'ayushi'.islower()
True
>>> '@yu$hi'.islower()
True
>>> '@YU$HI'.isupper()
True
>>> 'The Corpse Bride'.istitle()
True
22. Python中的pass语句是什么?
>>> def func(*args):
pass
>>>
>>> for i in range(7):
if i==3: break
print(i)
0
1
2
>>> for i in range(7):
if i==3: continue
print(i)
0
1
2
4
5
6
23. Python中的闭包是什么?
>>> def A(x):
def B():
print(x)
return B
>>> A(7)()
7
24. 解释一下Python中的//,%和 ** 运算符
>>> 7//2
3
>>> 2**10
1024
>>> 13%7
6
>>> 3.5%1.5
0.5
25. 在Python中有多少种运算符?解释一下算数运算符。
>>> 7+8
15
>>> 7-8
-1
>>> 7*8
56
>>> 7/8
0.875
>>> 1/1
1.0
26. 解释一下Python中的关系运算符
1.小于号(<),如果左边的值较小,则返回True。
>>> 'hi'<'Hi'
False
>>> 1.1+2.2>3.3
True
>>> 3.0<=3
True
>>> True>=False
True
>>> {1,3,2,2}=={1,2,3}
True
>>> True!=0.1
True
>>> False!=0.1
True
27. 解释一下Python中的赋值运算符
>>> a=7
>>> a+=1
>>> a
8
>>> a-=1
>>> a
7
>>> a*=2
>>> a
14
>>> a/=2
>>> a
7.0
>>> a**=2
>>> a
49
>>> a//=3
>>> a
16.0
>>> a%=4
>>> a
0.0
28. 解释一下Python中的逻辑运算符
>>> False and True
False
>>> 7<7 or True
True
>>> not 2==2
False
29. 解释一下Python中的成员运算符
>>> 'me' in 'disappointment'
True
>>> 'us' not in 'disappointment'
True
30. 解释一下Python中的身份运算符
>>> 10 is '10'
False
>>> True is not False
True
31. 讲讲Python中的位运算符
>>> 0b110 & 0b010
2
>>> 3|2
3
>>> 3^2
1
>>> ~2
-3
>>> 1<<2
4
>>> 4>>2
1
32. 在Python中如何使用多进制数字?
>>> int(0b1010)
10
>>> bin(0xf)
‘0b1111’
>>> oct(8)
‘0o10’
>>> hex(16)
‘0x10’
>>> hex(15)
‘0xf’
33. 怎样获取字典中所有键的列表?
>>> mydict={'a':1,'b':2,'c':3,'e':5}
>>> mydict.keys()
dict_keys(['a', 'b', 'c', 'e'])
34. 为何不建议以下划线作为标识符的开头
35. 怎样声明多个变量并赋值?
>>> a,b,c=3,4,5 #This assigns 3, 4, and 5 to a, b, and c respectively
>>> a=b=c=3 #This assigns 3 to a, b, and c
36. 元组的解封装是什么?
>>> mytuple=3,4,5
>>> mytuple
(3, 4, 5)
>>> x,y,z=mytuple
>>> x+y+z
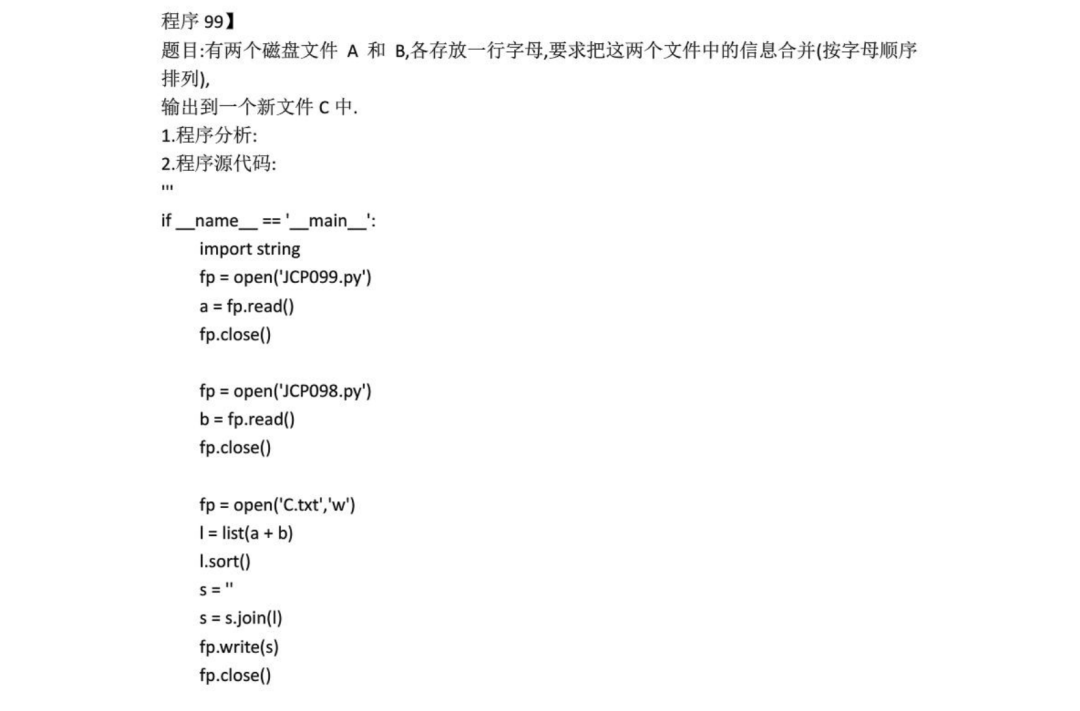
扫描二维码
获取更多精彩
印象python

点个在看你最好看

评论